C语言中的多线程编程技术
发布时间: 2024-03-29 12:07:57 阅读量: 36 订阅数: 43 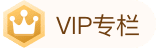
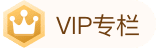
# 1. 多线程编程简介
1.1 什么是多线程编程
1.2 多线程编程的优势与应用场景
1.3 C语言中的多线程库
# 2. C语言中的线程创建与销毁
在多线程编程中,线程的创建和销毁是非常基础且重要的操作。本章将介绍C语言中如何创建和销毁线程,以及相关的函数的用法和注意事项。
### 2.1 线程的创建函数pthread_create的用法
在C语言中,线程的创建通过`pthread_create`函数来实现。下面是一个简单的示例代码,展示了如何使用`pthread_create`函数创建新线程。
```c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
void *thread_function(void *arg) {
int *value = (int *)arg;
printf("Hello from thread! The passed value is: %d\n", *value);
return NULL;
}
int main() {
pthread_t tid;
int value = 42;
pthread_create(&tid, NULL, thread_function, &value);
// 主线程等待新线程执行完毕
pthread_join(tid, NULL);
printf("Main thread is done!\n");
return 0;
}
```
**代码说明:**
- `pthread_create`函数用于创建一个新线程,参数依次为线程标识符、线程属性、线程函数和传递给线程函数的参数。
- 在示例中,新线程执行`thread_function`函数,并打印传递过来的值。
- 主线程使用`pthread_join`函数等待新线程执行完毕。
### 2.2 线程的退出与资源回收pthread_exit和pthread_join
在多线程编程中,线程的退出和资源回收是必不可少的操作。C语言提供了`pthread_exit`和`pthread_join`函数来实现线程的退出和资源回收。
```c
#include <stdio.h>
#include <pthread.h>
void *thread_function(void *arg) {
printf("Hello from thread!\n");
pthread_exit(NULL);
}
int main() {
pthread_t tid;
pthread_create(&tid, NULL, thread_function, NULL);
// 主线程等待新线程执行完毕
pthread_join(tid, NULL);
printf("Main thread is done!\n");
return 0;
}
```
**代码说明:**
- 在`thread_function`函数中,线程打印消息后调用`pthread_exit`函数退出。
- 主线程在调用`pthread_join`函数等待新线程执行完毕后才继续执行。
通过学习以上内容,我们了解了如何在C语言中创建和销毁线程,以及如何合理地进行线程的退出和资源回收,这是多线程编程中的基础知识。
# 3. 线程同步与互斥
在多线程编程中,线程之间的同步是非常重要的。当多个线程同时访问共享资源时,可能会出现竞态条件(Race Condition)和数据不一致的问题。为了避免这些问题,需要使用线程同步技术来确保线程的安全性和正确性。
#### 3.1 线程同步的概念与需求
线程同步是指多个线程按照一定的顺序来访问共享资源,以避免数据竞争和不一致的情况发生。在实际编程中,常用的线程同步技术包括互斥量(Mutex)、条件变量(Condition Variable)和信号量(Semaphore)等。
#### 3.2 互斥量(Mutex)的使用
互斥量是最常用的线程同步技术之一,它可以确保在同一时刻只有一个线程访问共享资源。在C语言中,可以使用`pthread_mutex_t`类型的变量来创建和管理互斥量。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
int shared_data = 0;
void* thread_function(void* arg) {
pthread_mutex_lock(&mutex);
shared_data++;
printf("Thread updated shared data to: %d\n", shared_data);
pthread_mutex_unlock(&mutex);
pthread_exit(NULL);
}
int main() {
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
pthread_create(&tid1, NULL, thread_function, NULL);
pthread_create(&tid2, NULL, thread_function, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
```
**代码说明**:
- 在主函数中创建了两个线程,它们会同时访问`shared_data`共享资源。
- 在线程函数中,使用`pthread_mutex_lock`和`pthread_mutex_unlock`来保护临界区,确保共享资源的安全访问。
#### 3.3 条件变量(Condition Vari
0
0
相关推荐







