opencv 连接骨架
时间: 2023-11-28 16:03:37 浏览: 194
根据提供的引用内容,似乎是在讨论图像处理中的骨架提取问题。下面是使用OpenCV进行骨架提取的方法:
1. 读取图像并将其转换为灰度图像
```python
import cv2
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 对灰度图像进行二值化处理
```python
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
3. 对二值化图像进行反色处理
```python
thresh = cv2.bitwise_not(thresh)
```
4. 对反色后的图像进行距离变换
```python
dist_transform = cv2.distanceTransform(thresh, cv2.DIST_L2, 5)
```
5. 对距离变换后的图像进行二值化处理
```python
ret, sure_fg = cv2.threshold(dist_transform, 0.7*dist_transform.max(), 255, 0)
```
6. 对二值化后的图像进行膨胀操作
```python
kernel = np.ones((3,3),np.uint8)
sure_fg = cv2.dilate(sure_fg,kernel,iterations=3)
```
7. 对膨胀后的图像进行距离变换
```python
dist_transform = cv2.distanceTransform(sure_fg, cv2.DIST_L2, 5)
```
8. 对距离变换后的图像进行二值化处理
```python
ret, sure_fg = cv2.threshold(dist_transform, 0.7*dist_transform.max(), 255, 0)
```
9. 对二值化后的图像进行反色处理
```python
sure_fg = cv2.bitwise_not(sure_fg)
```
10. 对反色后的图像进行骨架提取
```python
size = np.size(sure_fg)
skel = np.zeros(sure_fg.shape,np.uint8)
element = cv2.getStructuringElement(cv2.MORPH_CROSS,(3,3))
done = False
while(not done):
eroded = cv2.erode(sure_fg,element)
temp = cv2.dilate(eroded,element)
temp = cv2.subtract(sure_fg,temp)
skel = cv2.bitwise_or(skel,temp)
sure_fg = eroded.copy()
zeros = size - cv2.countNonZero(sure_fg)
if zeros==size:
done = True
```
以上就是使用OpenCV进行骨架提取的方法。需要注意的是,这里的骨架提取是基于二值化图像的,因此需要先对图像进行二值化处理。
阅读全文
相关推荐








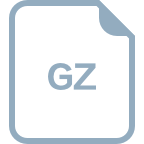









