用python编写一个程序,有一个列表中有四个字符串,请编写程序统计里面每一个字母出现的次数
时间: 2024-10-16 11:10:52 浏览: 29
你可以使用Python的内置模块`collections`中的`Counter`来统计列表中每个字母出现的次数。这里是一个简单的示例:
```python
from collections import Counter
# 定义包含四个字符串的列表
string_list = ['hello', 'world', 'python', 'coding']
# 合并所有字符串成为一个大字符串,因为Counter默认统计的是字符
merged_string = ''.join(string_list)
# 使用Counter计算字符频率
char_count = Counter(merged_string.lower())
# 打印结果
for char, count in char_count.items():
print(f"字母'{char}': {count}")
# 或者如果你想分别对每个字符串计数,可以遍历列表并独立统计
# for string in string_list:
# char_count_string = Counter(string.lower())
# print(f"{string}: {char_count_string}")
```
在这个例子中,我们将所有的字符串合并成一个大字符串,并将所有字母转为小写(因为大写字母会被视为不同的字符),然后用`Counter`进行计数。结果会是一个字典,其中键是字符,值是该字符出现的次数。
相关问题
python 编写一个函数,参数为一串明文密码字符串,返回值为字符串长度以及字符串里面的大写字母、小写字母,和数字的个数总共四个数字
好的,以下是 Python 代码实现:
```python
def analyze_password(password):
length = len(password)
upper_count = sum(1 for c in password if c.isupper())
lower_count = sum(1 for c in password if c.islower())
digit_count = sum(1 for c in password if c.isdigit())
return f"{length}{upper_count}{lower_count}{digit_count}"
```
这个函数接收一个字符串参数 `password`,返回一个字符串,字符串中依次包含密码长度、大写字母个数、小写字母个数、数字个数,共四个数字。函数内部使用了 Python 的字符串方法来统计各种字符的个数,并使用了 f-string 来拼接返回字符串。
题目描述 编写程序,输入一个字符串,用一个列表来保存字符串中元素的个数,其中第一个元素为大写字母个数,第二个元素为小写字母个数,第三元素为数字个数,第四个元素为其他字符个数。 输入 一个字符串 输出 包含各种元素个数的列表用python实现
好的,这是一个简单的程序,您可以参考以下代码:
```python
input_str = input("请输入一个字符串:")
element_count = [0, 0, 0, 0]
for char in input_str:
if char.isupper():
element_count[0] += 1
elif char.islower():
element_count[1] += 1
elif char.isdigit():
element_count[2] += 1
else:
element_count[3] += 1
print("大写字母个数:", element_count[0])
print("小写字母个数:", element_count[1])
print("数字个数:", element_count[2])
print("其他字符个数:", element_count[3])
```
这个程序首先接收用户从键盘输入的一个字符串,并将其赋值给变量input_str。然后定义一个长度为4的列表element_count,用于分别保存大写字母个数、小写字母个数、数字个数和其他字符个数。接下来使用for循环遍历字符串中的每个字符,根据字符的类型(大写字母、小写字母、数字、其他字符)分别将对应的元素计数加1。最后输出element_count列表中各个元素的值,即为字符串中各种元素的个数。
阅读全文
相关推荐
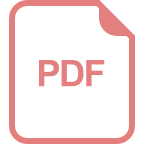
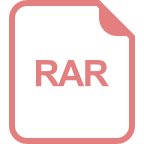
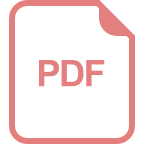













