数据结构 算术表达式求值 C++ 栈方法
时间: 2024-04-29 15:15:29 浏览: 80
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int evaluateExpression(string expression) {
stack<int> operandStack;
stack<char> operatorStack;
istringstream tokens(expression);
string token;
const string operators = "+-*/";
while (tokens >> token) {
if (token[0] == '+' || token[0] == '-') {
while (!operatorStack.empty() && (operatorStack.top() == '+' || operatorStack.top() == '-' ||
operatorStack.top() == '*' || operatorStack.top() == '/')) {
processAnOperator(operandStack, operatorStack);
}
operatorStack.push(token[0]);
} else if (token[0] == '*' || token[0] == '/') {
while (!operatorStack.empty() && (operatorStack.top() == '*' || operatorStack.top() == '/')) {
processAnOperator(operandStack, operatorStack);
}
operatorStack.push(token[0]);
} else if (token[0] == '(') {
operatorStack.push('(');
} else if (token[0] == ')') {
while (operatorStack.top() != '(') {
processAnOperator(operandStack, operatorStack);
}
operatorStack.pop();
} else {
operandStack.push(stoi(token));
}
}
while (!operatorStack.empty()) {
processAnOperator(operandStack, operatorStack);
}
return operandStack.top();
}
void processAnOperator(stack<int>& operandStack, stack<char>& operatorStack) {
char op = operatorStack.top();
operatorStack.pop();
int op1 = operandStack.top();
operandStack.pop();
int op2 = operandStack.top();
operandStack.pop();
if (op == '+') {
operandStack.push(op2 + op1);
} else if (op == '-') {
operandStack.push(op2 - op1);
} else if (op == '*') {
operandStack.push(op2 * op1);
} else if (op == '/') {
operandStack.push(op2 / op1);
}
}
int main() {
string expression = "3 + 4 * 5";
cout << "The result of the expression is: " << evaluateExpression(expression) << endl;
return 0;}
```
阅读全文
相关推荐
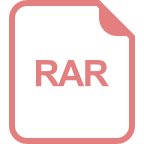
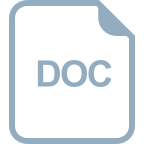
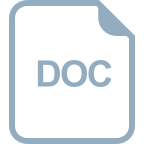
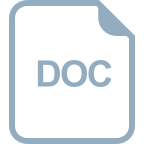
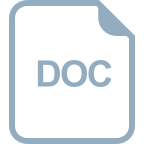
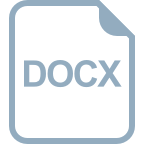
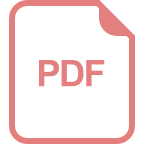
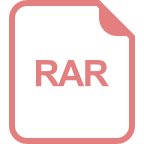


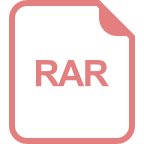
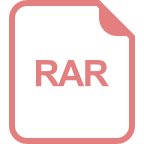
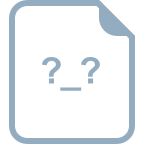
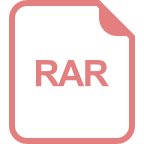
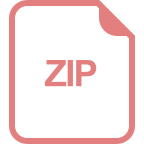