如何利用UICollectionView和自定义UICollectionViewLayout实现横向瀑布流布局?请提供详细的代码示例和关键步骤。
时间: 2024-11-21 15:44:31 浏览: 10
要在iOS中使用UICollectionView实现自定义的横向瀑布流布局,首先需要创建一个继承自UICollectionViewLayout的自定义布局类,例如WaterfallFlowLayout。这个类将负责管理行或列的宽度以及布局的计算。以下是具体的步骤和代码示例:
参考资源链接:[iOS UICollectionView 实现横向瀑布流布局教程](https://wenku.csdn.net/doc/eyta39i961?spm=1055.2569.3001.10343)
1. 创建自定义布局类WaterfallFlowLayout,并实现必要的方法。例如,计算单元格位置的`layoutAttributesForElements(in:)`和`layoutAttributesForItem(at:)`方法。
```swift
class WaterfallFlowLayout: UICollectionViewLayout {
// 定义布局属性,如列数,间距等
override func layoutAttributesForElements(in rect: CGRect) -> [UICollectionViewLayoutAttributes]? {
// 遍历所有cell,计算并返回布局属性
}
override func layoutAttributesForItem(at indexPath: IndexPath) -> UICollectionViewLayoutAttributes? {
// 根据indexPath返回单元格的布局属性
}
// 其他必要的方法,如计算总大小等
}
```
2. 实现宽度计算代理协议,并在代理方法中根据单元格内容动态计算宽度。
```swift
protocol WaterfallFlowLayoutDelegate {
func waterfallFlowLayout(_ layout: WaterfallFlowLayout, widthForItemAt indexPath: IndexPath) -> CGFloat
}
class YourViewController: UIViewController, UICollectionViewDelegate, WaterfallFlowLayoutDelegate {
func waterfallFlowLayout(_ layout: WaterfallFlowLayout, widthForItemAt indexPath: IndexPath) -> CGFloat {
// 返回对应单元格的宽度
}
}
```
3. 在UICollectionView的数据源方法中,使用自定义布局,并设置其代理。
```swift
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout) -> UICollectionViewLayout {
if let flowLayout = collectionViewLayout as? WaterfallFlowLayout {
flowLayout.delegate = self
}
return flowLayout
}
```
4. 实现UICollectionViewDelegateFlowLayout协议的方法,如间距设置等。
```swift
extension YourViewController: UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAt section: Int) -> CGFloat {
return 10.0
}
// 其他间距和间隔的设置
}
```
通过以上步骤,你可以利用UICollectionView实现一个横向瀑布流布局。建议深入学习UICollectionView的工作原理以及自定义布局的高级用法,这样可以更灵活地处理复杂的布局需求。为了更好地理解和掌握UICollectionView的布局技术,你可以参考这份资料:《iOS UICollectionView 实现横向瀑布流布局教程》。这份资源不仅能够帮助你解决当前的问题,还会提供更深层次的布局设计知识,为你的iOS开发之路增添更多色彩。
参考资源链接:[iOS UICollectionView 实现横向瀑布流布局教程](https://wenku.csdn.net/doc/eyta39i961?spm=1055.2569.3001.10343)
阅读全文
相关推荐
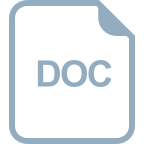
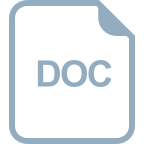
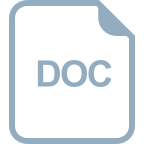

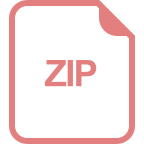
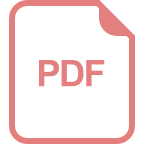
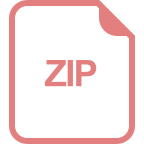
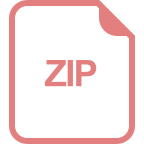
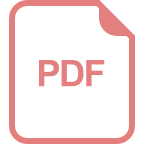
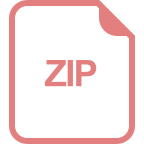
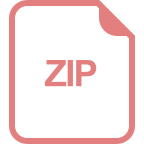
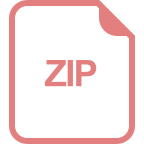
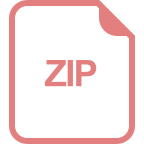
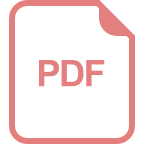
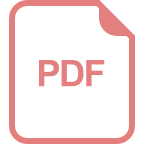
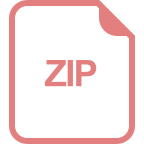
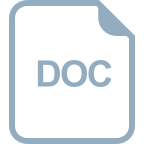
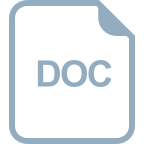