Configure pins as * Analog * Input * Output * EVENT_OUT * EXTI */ static void MX_GPIO_Init(void) { GPIO_InitTypeDef GPIO_InitStruct; /* GPIO Ports Clock Enable */ //__HAL_RCC_GPIOH_CLK_ENABLE(); __HAL_RCC_GPIOC_CLK_ENABLE(); //__HAL_RCC_GPIOA_CLK_ENABLE(); __HAL_RCC_GPIOD_CLK_ENABLE(); __HAL_RCC_GPIOB_CLK_ENABLE(); /*Configure GPIO pin Output Level */ HAL_GPIO_WritePin(LEDR_OUT_PD3_GPIO_Port, LEDR_OUT_PD3_Pin, GPIO_PIN_SET); /*Configure GPIO pin Output Level */ //HAL_GPIO_WritePin(GPIOB, RS485_RE_OUT_PB8_Pin|RS485_SE_OUT_PB9_Pin, GPIO_PIN_RESET); /*Configure GPIO pin : LEDR_OUT_PD3_Pin */ GPIO_InitStruct.Pin = LEDR_OUT_PD3_Pin; GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; GPIO_InitStruct.Pull = GPIO_PULLUP; GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; HAL_GPIO_Init(LEDR_OUT_PD3_GPIO_Port, &GPIO_InitStruct); /*Configure GPIO pins : RS485_RE_OUT_PB8_Pin RS485_SE_OUT_PB9_Pin */ GPIO_InitStruct.Pin = RS485_RE_OUT_PB8_Pin|RS485_SE_OUT_PB9_Pin; GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; GPIO_InitStruct.Pull = GPIO_PULLUP; GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; HAL_GPIO_Init(GPIOB, &GPIO_InitStruct); } /* USER CODE BEGIN 4 */ /* USER CODE END 4 */ /** * @brief This function is executed in case of error occurrence. * @param file: The file name as string. * @param line: The line in file as a number. * @retval None */ void _Error_Handler(char *file, int line) { /* USER CODE BEGIN Error_Handler_Debug */ /* User can add his own implementation to report the HAL error return state */ while(1) { } /* USER CODE END Error_Handler_Debug */
时间: 2023-09-16 12:16:11 浏览: 298
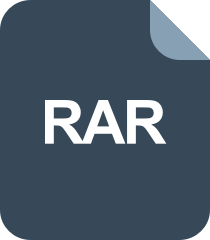
output_example.rar_FFmpeg解码_ffmpeg_ffmpeg 编译_output exmple_outpu
这是用于初始化GPIO引脚的函数。在该函数中,首先使能了GPIOC、GPIOD和GPIOB端口的时钟。然后,配置了LEDR_OUT_PD3_Pin引脚为输出模式,初始电平为高电平。接着,配置了RS485_RE_OUT_PB8_Pin和RS485_SE_OUT_PB9_Pin引脚为输出模式,初始电平为高电平。最后,定义了一个错误处理函数_Error_Handler,当发生错误时会进入该函数进行处理。
需要注意的是,该函数中有一段注释掉的代码,可能是之前的配置代码被注释掉了。
阅读全文