STM32 Microcontroller IO Port Programming Secrets: In-depth Analysis of GPIO Configuration, Reading/Writing, and Interrupts, Mastering Microcontroller Input/Output
发布时间: 2024-09-14 15:39:51 阅读量: 47 订阅数: 22 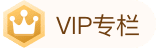
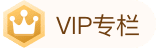
# STM32 Microcontroller IO Port Programming: A Comprehensive Guide to GPIO Configuration, Reading/Writing, and Interrupts, Mastering MCU Input/Output
## 1. Basics of STM32 Microcontroller IO Ports
STM32 microcontroller IO ports serve as critical interfaces for interaction with the outside world, handling data input/output and controlling peripheral devices. This chapter will cover the foundational knowledge of STM32 microcontroller IO ports, including the classification of IO ports, pin functionalities, and IO port registers.
### 1.1 Classification of IO Ports
STM32 microcontroller IO ports are divided into general-purpose IO ports and special-function IO ports. General-purpose IO ports can serve as universal input/output pins, whereas special-function IO ports have specific functionalities, such as timers, serial ports, I2C, etc.
### 1.2 Pin Functionalities
Each IO port pin can be configured for various functions, such as input, output, push-pull output, open-drain output, etc. These functionalities are configured through the IO port registers.
## 2. GPIO Configuration and Read/Write Operations
### 2.1 Principles of GPIO Configuration
GPIO configuration is fundamental to STM32 microcontroller IO port programming as it determines the IO port's input/output mode, speed, and strength attributes.
#### 2.1.1 Selection of GPIO Modes
The selection of GPIO modes dictates the IO port's input/output direction. STM32 microcontrollers offer a variety of GPIO modes, including:
- **Input Mode (Input)**: The IO port serves as an input port, reading external signals.
- **Output Mode (Output)**: The IO port serves as an output port, outputting level signals.
- **Push-Pull Output Mode (Push-Pull Output)**: The IO port can output both high and low levels.
- **Open-Drain Output Mode (Open-Drain Output)**: The IO port can only output a low level and requires an external pull-up resistor to output a high level.
- **Analog Input Mode (Analog Input)**: The IO port acts as an analog input port, capable of reading analog signals.
GPIO mode selection is configured through the register `GPIOx_MODER`, where `x` denotes the GPIO port number (A, B, C, D, E, F, G, H).
```c
#define GPIOA_MODER ((volatile uint32_t*)0x***)
// Configure GPIOA bit 0 to input mode
*GPIOA_MODER &= ~(3 << 0);
*GPIOA_MODER |= (0 << 0);
```
#### 2.1.2 GPIO Speed and Strength Settings
GPIO speed and strength settings determine the driving capability and interference resistance of the IO port. STM32 microcontrollers offer various speed and strength settings, including:
- **Speed**:
- Low-speed (2 MHz)
- Medium-speed (10 MHz)
- High-speed (50 MHz)
- Very high-speed (100 MHz)
- **Strength**:
- Weak strength
- Medium strength
- Strong strength
GPIO speed and strength settings are configured through the registers `GPIOx_OSPEEDR` and `GPIOx_PUPDR`, where `x` denotes the GPIO port number (A, B, C, D, E, F, G, H).
```c
#define GPIOA_OSPEEDR ((volatile uint32_t*)0x***)
#define GPIOA_PUPDR ((volatile uint32_t*)0x4002000C)
// Configure GPIOA bit 0 to high-speed, strong-strength output
*GPIOA_OSPEEDR |= (3 << 0);
*GPIOA_PUPDR |= (1 << 0);
```
### 2.2 GPIO Read/Write Operations
GPIO read/write operations are the core of STM32 microcontroller IO port programming, facilitating the input/output of data.
#### 2.2.1 GPIO Input Reading
GPIO input reading is performed through the register `GPIOx_IDR`, where `x` denotes the GPIO port number (A, B, C, D, E, F, G, H).
```c
#define GPIOA_IDR ((volatile uint32_t*)0x***)
// Read the input level of GPIOA bit 0
uint32_t input_value = *GPIOA_IDR & (1 << 0
```
0
0
相关推荐








