Detailed Explanation of STM32 Microcontroller I2C Communication: Elaborating on the I2C Protocol, Configuration, and Application, Mastering Low-Speed Data Transfer
发布时间: 2024-09-14 15:47:48 阅读量: 38 订阅数: 40 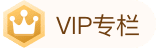
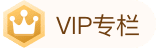
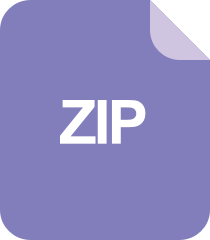
How-to-use-the-Arduino-Wire-library:关于使用 Arduino Wire 库时常见错误的 Wiki
# 1. Overview of STM32 MCU I2C Communication
I2C (Inter-Integrated Circuit) is a serial communication protocol widely used in embedded systems to connect microcontrollers with peripheral devices. The STM32 microcontroller comes equipped with an I2C interface, enabling communication with various I2C devices.
I2C communication operates in a master-slave mode, with a master device controlling the communication process and multiple slave devices responding to the master's requests. The I2C bus utilizes two lines: the Serial Data Line (SDA) and the Serial Clock Line (SCL). The master device sends and receives data via the SDA line, while the SCL line provides the clock signal.
I2C communication has the following features:
- Cost-effective and low power consumption
- Supports multiple masters and multiple slaves
- Configurable data transfer rates
- Addressing functionality for easy identification of different slave devices
# 2. Detailed Explanation of the I2C Protocol
### 2.1 Basic Principles of the I2C Protocol
I2C (Inter-Integrated Circuit) is a serial communication protocol used for data transfer between integrated circuits (ICs). It is a half-duplex communication protocol, meaning devices can send and receive data on the same bus simultaneously. The features of the I2C protocol include:
- **Low speed:** I2C protocol transfer rates are typically between 100 kbps and 1 Mbps.
- **Multi-master capability:** Multiple master devices can be connected to the I2C bus, but only one master can control the bus at a time.
- **Slave device addressing:** Each slave device has a unique 7-bit or 10-bit address, and the master device selects which slave device to communicate with by sending the address.
- **Data transfer:** Data is transmitted in 8-bit bytes, followed by an ACK (acknowledgment) or NACK (non-acknowledgment) signal.
### 2.2 I2C Protocol Data Transfer Format
The I2C protocol data transfer format includes the following parts:
- **Start bit:** Sent by the master device, indicating the start of data transfer.
- **Slave address:** Sent by the master device to specify the slave device to communicate with.
- **Read/Write bit:** Sent by the master device to indicate whether it intends to read or write data.
- **Data:** Exchanged between the master device and the slave device.
- **Stop bit:** Sent by the master device, indicating the end of data transfer.
### 2.3 I2C Protocol Addressing and Data Transfer Process
The I2C protocol addressing and data transfer process is as follows:
1. **The master device sends the start bit.**
2. **The master device sends the slave address.**
3. **The slave device responds and sends an ACK signal.**
4. **The master device sends the read/write bit.**
5. **The slave device responds again and sends an ACK signal.**
6. **The master device and the slave device exchange data.**
7. **The master device sends the stop bit.**
**Code Example:**
```c
// Send start bit
I2C_SendStart(I2C1);
// Send 7-bit slave address
I2C_Send7bitAddress(I2C1, 0x50, I2C_Direction_Transmitter);
// Wait for slave response
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_RECEIVER_MODE_SELECTED));
// Send data
I2C_SendData(I2C1, 0x00);
// Wait for data transmission to complete
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
// Send stop bit
I2C_SendStop(I2C1);
```
**Code Logic Analysis:**
This code segment demonstrates how to use the I2C protocol to send start bit, slave address, data, and stop bit.
- `I2C_SendStart(I2C1)`: Sends the start bit.
- `I2C_Send7bitAddress(I2C1, 0x50, I2C_Direction_Transmitter)`: Sends the 7-bit slave address (0x50) and read/write bit (transmitter).
- `while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_RECEIVER_MODE_S
0
0
相关推荐
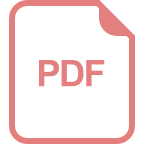
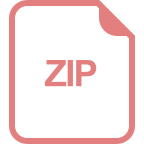
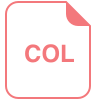
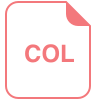
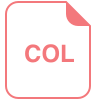
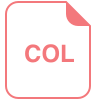
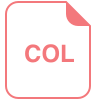
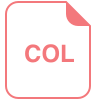
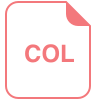