In-depth Analysis of STM32 Microcontroller Interrupt Mechanism: From Interrupt Vector Table to Interrupt Priority, Fully Mastering the Interrupt Mechanism
发布时间: 2024-09-14 15:38:46 阅读量: 47 订阅数: 28 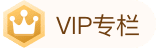
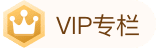
# In-depth Analysis of STM32 MCU Interrupt Mechanism: From Interrupt Vector Table to Priority, Mastering the Interrupt Mechanism
## 1. Fundamentals of Interrupt Mechanism**
The interrupt mechanism is a crucial aspect of microcontrollers that allows the pausing and resuming of program execution in response to external events or internal conditions. In STM32 microcontrollers, this mechanism is managed by specialized hardware modules, providing efficient and configurable interrupt handling capabilities.
**Classification of Interrupts:**
***External Interrupts:** Triggered by signals on external pins, such as button presses or sensor inputs.
***Internal Interrupts:** Triggered by internal events, such as timer overflows or completed data transmissions.
**Interrupt Handling Process:**
Upon the occurrence of an interrupt, the STM32 microcontroller halts the currently executing program and jumps to the specified Interrupt Service Routine (ISR) in the interrupt vector table. The ISR is responsible for handling the interrupt event, executing necessary operations, and then returning to the main program.
## 2. Interrupt Vector Table and Interrupt Service Routines
### 2.1 Structure of the Interrupt Vector Table
The interrupt vector table is an array that stores the entry addresses of interrupt service routines. The STM32 microcontroller's interrupt vector table is located at memory address 0x*** and contains 256 32-bit entry addresses. Each entry corresponds to an interrupt source. When this interrupt source is triggered, the CPU will jump to the corresponding entry address to execute the interrupt service routine.
The structure of the interrupt vector table is as follows:
| Interrupt Source | Entry Address |
|---|---|
| Reset | 0x*** |
| NMI | 0x*** |
| Hard Fault | 0x0000 000C |
| MemManage | 0x*** |
| BusFault | 0x*** |
| UsageFault | 0x*** |
| SVC | 0x0000 001C |
| DebugMon | 0x*** |
| PendSV | 0x*** |
| SysTick | 0x*** |
| ... | ... |
### 2.2 Writing Interrupt Service Routines
An interrupt service routine is the code segment that responds to an interrupt request. The writing of interrupt service routines must follow these rules:
- Interrupt service routines must be declared with `__attribute__((interrupt))` to indicate that the function is an interrupt handler.
- Interrupt service routines must have a parameter, which is the interrupt source number.
- Interrupt service routines must be declared with `__attribute__((naked))` to indicate that the function has no prologue or epilogue.
- Interrupt service routines must use assembly instructions `push` and `pop` to save and restore registers.
- Interrupt service routines must use the assembly instruction `bl` to call C language functions.
Here is an example of an interrupt service routine:
```c
__attribute__((interrupt))
void USART1_IRQHandler(void)
{
__attribute__((naked))
{
// Save registers
push({r0, r1, r2, r3, r12, lr})
// Call C language function to handle interrupt
bl USART1_IRQHandler_C
// Restore registers
pop({r0, r1, r2, r3, r12, lr})
// Return to the interrupt vector table
bx lr
}
}
```
In the C language function `USART1_IRQHandler_C`, the specific interrupt handling logic can be written.
## 3.1 Setting Interrupt Priority
STM32 microcontroller interrupt priorities are divided into 16 levels, with level 0 being the highest priority and level 15 being the lowest. Interrupt priorities can be set via the NVIC registers.
**NVIC Register Structure**
```c
typedef struct {
__IO uint32_t ISER[8]; /* Interrupt Set Enable Register */
__IO uint32_t ICER[8]; /* Interrupt Clear Enable Register */
__IO uint32_t ISPR[8]; /* Interrupt Set Pending Register */
__IO uint32_t ICPR[8]; /* Interrupt Clear Pending Register */
__IO uint32_t IABR[8]; /* Interrupt Active Bit Register */
__IO uint8_t IP[80]; /* Interrupt Priority Register (80 interrupts) */
__IO uint32_t STIR; /* Software Trigger Interrupt Register */
} NVIC_TypeDef;
```
**Setting Interrupt Priority Code**
```c
/* Set interrupt priority */
NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority);
/* Get interrupt priority */
uint32_t NVIC_GetPriority(IRQn_Type IRQn);
```
**Parameter Description**
***IRQn:** Interrupt number
***priority:** Priority level, ranging from 0 to 15
**Code Logic**
* The `NVIC_SetPriority()` function sets the interrupt priority of the specified interrupt number to the specified priority.
* The `NVIC_GetPriority()` function retrieves the interrupt priority of the specified interrupt number.
### 3.2 Interrupt Nesting Management
The STM32 microcontroller supports interrupt nesting, meaning a lower priority interrupt can be interrupted by a higher priority one during its handling. Interrupt nesting can be managed via the NVIC registers.
**NVIC Register Structure**
```c
typedef struct {
__IO uint32_t ISER[8]; /* Interrupt Set Enable Register */
__IO uint32_t ICER[8]; /* Interrupt Clear Enable Register */
__IO uint32_t ISPR[8]; /* Interrupt Set Pending Register */
__IO uint32_t ICPR[8]; /* Interrupt Clear Pending Register */
__IO uint32_t IABR[8]; /* Interrupt Active Bit Register */
__IO uint8_t IP[80]; /* Interrupt Priority Register (80 interrupts) */
__IO uint32_t STIR; /* Software Trigger Interrupt Register */
__IO uint32_t ICER[4]; /* Interrupt Clear Enable Register */
__IO uint32_t ISPR[4]; /* Interrupt Set Pending Register */
__IO uint32_t ICPR[4]; /* Interrupt Clear Pending Register */
__IO uint32_t IABR[4]; /* Interrupt Active Bit Register */
__IO uint8_t IP[24]; /* Interrupt Priority Register (24 interrupts) */
} NVIC_Type;
```
**Interrupt Nesting Management Code**
```c
/* Set interrupt nesting */
NVIC_SetPriorityGrouping(uint32_t priorityGrouping);
/* Get interrupt nesting */
uint32_t NVIC_GetPriorityGrouping();
```
**Parameter Description**
***priorityGrouping:** Priority grouping, ranging from 0 to 7
**Code Logic**
* The `NVIC_SetPriorityGrouping()` function sets the priority grouping for interrupt nesting. The priority grouping determines the allocation of interrupt priorities and subpriorities.
* The `NVIC_GetPriorityGrouping()` function retrieves the priority grouping for interrupt nesting.
## 4. Interrupt Response and Service
### 4.1 Interrupt Response Process
When an interrupt event occurs, the STM32 microcontroller executes the following interrupt response process:
1. **Interrupt Request Detection:** The interrupt controller detects an interrupt request signal and determines the interrupt source.
2. **Interrupt Vector Table Lookup:** The interrupt controller looks up the corresponding interrupt service routine entry address in the interrupt vector table based on the interrupt source address.
3. **Program Counter Update:** The program counter (PC) is updated to the interrupt service routine entry address, beginning the execution of the interrupt service routine.
4. **Interrupt Masking:** The interrupt controller masks the current interrupt source to prevent the same interrupt from triggering again during the execution of the interrupt service routine.
5. **Interrupt Flag Clearing:** The interrupt controller clears the interrupt source's interrupt flag bit, indicating that the interrupt event has been handled.
### 4.2 Interrupt Service Process
The interrupt service routine is the code segment that responds to interrupt events and typically includes the following steps:
1. **Save Registers:** At the beginning of the interrupt service routine, save the register values of the current program state, including the program counter (PC), stack pointer (SP), and general-purpose registers (R0-R15).
2. **Handle Interrupt Event:** Depending on the interrupt source, execute the corresponding processing logic, such as reading input data, updating state variables, or sending data.
3. **Restore Registers:** At the end of the interrupt service routine, restore the previously saved register values to resume the program state before the interrupt.
4. **Interrupt Return:** Execute an interrupt return instruction (RET) to update the program counter to the address before the interrupt occurred, continuing the execution of the main program.
### Code Example
The following code example demonstrates an interrupt service routine:
```c
// Interrupt service routine
void SysTick_Handler(void) {
// Save registers
__asm volatile("push {r0-r3, r12}");
// Handle interrupt event
// ...
// Restore registers
__asm volatile("pop {r0-r3, r12}");
// Interrupt return
__asm volatile("bx lr");
}
```
### Flowchart
The following diagram illustrates the interrupt response and service process:
```mermaid
sequenceDiagram
participant MCU
participant Interrupt Controller
MCU->Interrupt Controller: Interrupt Request
Interrupt Controller->MCU: Interrupt Vector Table Lookup
MCU->Interrupt Controller: Program Counter Update
Interrupt Controller->MCU: Interrupt Masking
Interrupt Controller->MCU: Interrupt Flag Clearing
MCU->Interrupt Controller: Interrupt Service Routine Entry
MCU->Interrupt Controller: Register Saving
MCU->Interrupt Controller: Interrupt Event Handling
MCU->Interrupt Controller: Register Restoring
MCU->Interrupt Controller: Interrupt Return
```
## 5. Applications of Interrupt Mechanism in STM32 Microcontrollers
### 5.1 Application of External Interrupts
**5.1.1 Introduction to External Interrupts**
External interrupts are a mechanism by which STM32 microcontrollers receive external events through external pins. They allow the microcontroller to trigger interrupt responses upon detecting changes in external signals. External interrupts can be classified into two types: rising-edge triggered and falling-edge triggered.
**5.1.2 Configuration of External Interrupts**
Configuring external interrupts involves the following steps:
1. **Enable Clock:** Enable the clock for the GPIO port where the external interrupt pin is located.
2. **Configure GPIO Pin:** Set the external interrupt pin to input mode and select the trigger type (rising edge or falling edge).
3. **Configure NVIC:** Enable the external interrupt in the NVIC and set the priority.
**5.1.3 External Interrupt Service Routine**
The external interrupt service routine is a function that responds to external interrupt events. It typically includes the following steps:
1. **Read Interrupt Flag Bit:** Read the external interrupt flag bit to determine the pin that triggered the interrupt.
2. **Clear Interrupt Flag Bit:** Clear the external interrupt flag bit to allow subsequent interrupt events to trigger.
3. **Execute Interrupt Handling:** Perform operations related to the interrupt event.
### 5.2 Application of Timer Interrupts
**5.2.1 Introduction to Timer Interrupts**
Timer interrupts are periodic interrupts generated by the timer peripherals of STM32 microcontrollers. They allow the microcontroller to trigger interrupt responses at specific time intervals. Timer interrupts can be used to implement various functions, such as timing, counting, and pulse-width modulation.
**5.2.2 Configuration of Timer Interrupts**
Configuring timer interrupts involves the following steps:
1. **Enable Clock:** Enable the clock for the timer peripheral.
2. **Configure Timer:** Set the timer's operating mode, clock source, and count value.
3. **Configure NVIC:** Enable the timer interrupt in the NVIC and set the priority.
**5.2.3 Timer Interrupt Service Routine**
The timer interrupt service routine is a function that responds to timer interrupt events. It typically includes the following steps:
1. **Read Interrupt Flag Bit:** Read the timer interrupt flag bit to determine the timer that triggered the interrupt.
2. **Clear Interrupt Flag Bit:** Clear the timer interrupt flag bit to allow subsequent interrupt events to trigger.
3. **Execute Interrupt Handling:** Perform operations related to the timer interrupt event.
### 5.3 Application of Serial Communication Interrupts
**5.3.1 Introduction to Serial Communication Interrupts**
Serial communication interrupts are receive and transmit interrupts generated by the serial peripheral of STM32 microcontrollers. They allow the microcontroller to trigger interrupt responses when data is received or transmission is completed. Serial communication interrupts can be used to implement various functions, such as data transfer, debugging, and communication.
**5.3.2 Configuration of Serial Communication Interrupts**
Configuring serial communication interrupts involves the following steps:
1. **Enable Clock:** Enable the clock for the serial peripheral.
2. **Configure Serial Interface:** Set the serial interface's operating mode, baud rate, and data format.
3. **Configure NVIC:** Enable the serial communication interrupt in the NVIC and set the priority.
**5.3.3 Serial Communication Interrupt Service Routine**
The serial communication interrupt service routine is a function that responds to serial communication interrupt events. It typically includes the following steps:
1. **Read Interrupt Flag Bit:** Read the serial communication interrupt flag bit to determine the event that triggered the interrupt (receive or transmit).
2. **Clear Interrupt Flag Bit:** Clear the serial communication interrupt flag bit to allow subsequent interrupt events to trigger.
3. **Execute Interrupt Handling:** Perform operations related to the serial communication interrupt event, such as receiving data or transmitting data.
## 6. Optimization and Debugging of Interrupt Mechanism**
### 6.1 Interrupt Optimization Strategies
Interrupt optimization aims to improve interrupt response speed and system efficiency. Main strategies include:
- **Reduce the code volume in interrupt service routines:** Only execute necessary tasks and avoid redundant operations.
- **Use interrupt priorities:** Set interrupt priorities reasonably to ensure that important interrupts are responded to first.
- **Use interrupt nesting:** Allow high-priority interrupts to interrupt low-priority ones to increase response speed.
- **Use DMA (Direct Memory Access):** Offload data transfers from the CPU to the DMA controller to reduce CPU overhead.
- **Use interrupt grouping:** Group related interrupts for easier management and optimization.
### ***
***mon techniques include:
- **Use a debugger:** Set breakpoints, step through execution, and trace the interrupt response and service process.
- **Use a logic analyzer:** Capture interrupt signals and analyze timing and trigger conditions.
- **Use printf output:** Output debug information in the interrupt service routine to understand interrupt triggering and execution.
- **Use interrupt flag registers:** Check the interrupt flag registers to determine the interrupt source and status.
- **Use interrupt priority registers:** Check the interrupt priority settings to ensure they are correctly configured.
0
0
相关推荐
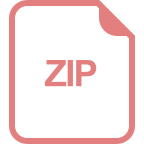
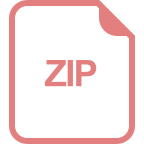
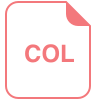
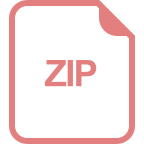
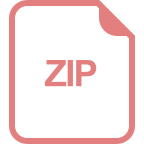
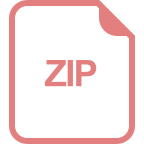
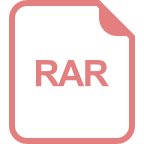
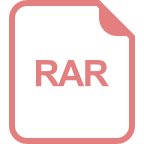
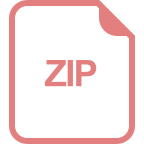