Comprehensive Guide to STM32 MCU Clock System: Elucidating Clock Sources, Dividers, and Clock Trees, Aiding in Mastering the Time Pulse of Microcontrollers
发布时间: 2024-09-14 15:37:51 阅读量: 37 订阅数: 40 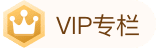
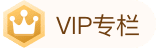
# 1. Overview of the Clock System**
The clock system is the critical module in a microcontroller that generates and distributes clock signals. These signals serve as the reference for executing instructions and processing data by the microcontroller. The STM32 microcontroller boasts a robust clock system with multiple clock sources and division options to meet various application requirements.
The clock system mainly consists of the following components:
- **Clock Sources:** Clock sources are the origin of clock signals, which can be internal (e.g., HSI, LSI) or external (e.g., HSE, LSE).
- **Dividers:** Dividers are used to divide the frequency of the clock source to produce clock signals of different frequencies.
- **Clock Tree:** The clock tree is a hierarchical structure that distributes clock signals from the clock sources to various peripherals of the microcontroller.
# 2. Clock Sources
The STM32 microcontroller's clock system has various clock sources to satisfy requirements across different scenarios. Clock sources are divided into internal and external sources.
### 2.1 Internal Clock Sources
Internal clock sources are integrated into the microcontroller and do not require external components.
#### 2.1.1 HSI
HSI (High-Speed Internal) is an RC oscillator inside the microcontroller, typically operating at 8MHz. HSI boasts fast startup and low power consumption but has lower accuracy.
```c
RCC->CR |= RCC_CR_HSION; // Enable HSI
```
#### 2.1.2 LSI
LSI (Low-Speed Internal) is another RC oscillator within the microcontroller, usually at 32kHz. LSI has higher accuracy than HSI but is slower to start up and consumes more power.
```c
RCC->CSR |= RCC_CSR_LSION; // Enable LSI
```
#### 2.1.3 HSE
HSE (High-Speed External) is an external crystal oscillator typically operating at 8MHz or 16MHz. HSE offers the highest precision but requires an external crystal component.
```c
RCC->CR |= RCC_CR_HSEON; // Enable HSE
```
### 2.2 External Clock Sources
External clock sources require external components to provide.
#### 2.2.1 LSE
LSE (Low-Speed External) is a 32.768kHz crystal oscillator outside the microcontroller. LSE has higher accuracy than LSI but consumes more power.
```c
RCC->BDCR |= RCC_BDCR_LSEON; // Enable LSE
```
#### 2.2.2 LFO
LFO (Low-Frequency Oscillator) is a 32kHz RC oscillator outside the microcontroller. LFO has lower accuracy than LSE but consumes less power.
```c
RCC->CSR |= RCC_CSR_LFOEN; // Enable LFO
```
**Clock Source Selection**
Different clock sources possess varying characteristics. When choosing, consider factors such as accuracy, power consumption, and cost for the application scenario. Typically, high accuracy and low power consumption clock sources are preferred.
# 3. Clock Division**
Clock division is a crucial aspect of the clock system, allowing it to divide high-frequency clock signals into multiple low-frequency signals to meet the requirements of various peripherals. The STM32 microcontroller offers various dividers, including PLLs (Phase-Locked Loops) and dividers, enabling flexible clock division.
### 3.1 PLL (Phase-Locked Loop)
A PLL is an analog circuit that can multiply or divide an input clock signal to create a new clock signal. The STM32 microcontroller integrates multiple PLLs, allowing for flexible clock division and multiplication.
#### 3.1.1 PLL Multiplication
The PLL multiplication feature can multiply the input clock signal to a higher frequency. The multiplication factor is set in the PLL register's MUL field. For example, if the input clock signal is 8MHz and the PLL multiplication factor is set to 10, the output clock signal from the PLL would be 80MHz.
```c
RCC->PLLCFGR |= RCC_PLLCFGR_PLLMULL10;
```
#### 3.1.2 PLL Division
The PLL division feature can divide the output clock signal of the PLL to a lower frequency. The division factor is set in the PLL register's DIV field. For instance, if the PLL output clock signal is 80MHz and the PLL division factor is set to 2, the output clock signal from the PLL would be 40MHz.
```c
RCC->PLLCFGR |= RCC_PLLCFGR_PLLDIV2;
```
### 3.2 Dividers
Dividers are digital circuits that divide an input clock signal into multiple lower-frequency signals. The STM32 microcontroller features multiple dividers, including AHB dividers, APB1 dividers, and APB2 dividers.
#### 3.2.1 AHB Divider
The AHB divider can divide the PLL output clock signal into an AHB bus clock signal. The AHB bus is the high-speed bus in the STM32 microcontroller, connecting high-speed peripherals such as DMA, SRAM, etc. The AHB division factor is set in the HPRE field of the RCC register. For example, if the PLL output clock signal is 80MHz and the AHB division factor is set to 2, the AHB bus clock signal would be 40MHz.
```c
RCC->CFGR |= RCC_CFGR_HPRE_DIV2;
```
#### 3.2.2 APB1 Divider
The APB1 divider can divide the AHB bus clock signal into an APB1 bus clock signal. The APB1 bus is the medium-speed bus in the STM32 microcontroller, connecting medium-speed peripherals like UART, I2C, etc. The APB1 division factor is set in the PPRE1 field of the RCC register. For example, if the AHB bus clock signal is 40MHz and the APB1 division factor is set to 2, the APB1 bus clock signal would be 20MHz.
```c
RCC->CFGR |= RCC_CFGR_PPRE1_DIV2;
```
#### 3.2.3 APB2 Divider
The APB2 divider can divide the AHB bus clock signal into an APB2 bus clock signal. The APB2 bus is the low-speed bus in the STM32 microcontroller, connecting low-speed peripherals such as GPIO, timers, etc. The APB2 division factor is set in the PPRE2 field of the RCC register. For example, if the AHB bus clock signal is 40MHz and the APB2 division factor is set to 4, the APB2 bus clock signal would be 10MHz.
```c
RCC->CFGR |= RCC_CFGR_PPRE2_DIV4;
```
# 4. Clock Tree
### 4.1 Clock Tree Structure
The clock tree is a vital part of the clock system, responsible for distributing clock signals from the clock source to various peripherals on the chip. The structure of the clock tree can be divided into three parts:
- **Clock Sources:** The root node of the clock tree, providing the source of the clock signals.
- **Dividers:** Used to divide the clock signals to produce different frequency clock signals.
- **Clock Outputs:** The leaf nodes of the clock tree, outputting the clock signals to various peripherals on the chip.
### 4.1.1 Clock Sources
Clock sources are the root nodes of the clock tree, providing the origin of the clock signals. The STM32 microcontroller supports multiple clock sources, including:
- **Internal Clock Sources:** HSI, LSI, HSE
- **External Clock Sources:** LSE, LFO
### 4.1.2 Dividers
Dividers are used to divide the clock signals to produce different frequencies. The STM32 microcontroller supports various dividers, including:
- **PLL:** A multiplication and division divider that can multiply or divide the clock signals.
- **AHB Divider:** Used to divide the AHB bus clock signal.
- **APB1 Divider:** Used to divide the APB1 bus clock signal.
- **APB2 Divider:** Used to divide the APB2 bus clock signal.
### 4.1.3 Clock Outputs
Clock outputs are the leaf nodes of the clock tree, outputting the clock signals to various peripherals on the chip. The STM32 microcontroller supports multiple clock outputs, including:
- **APB1 Clock Output:** Used to provide clock signals to peripherals on the APB1 bus.
- **APB2 Clock Output:** Used to provide clock signals to peripherals on the APB2 bus.
- **AHB Clock Output:** Used to provide clock signals to peripherals on the AHB bus.
- **Other Clock Outputs:** Used to provide clock signals to specific peripherals, such as ADC, DAC, USB, etc.
### 4.2 Clock Tree Configuration
The clock tree configuration can be set through the RCC (Reset and Clock Control) register. The RCC register provides control over clock sources, dividers, and clock outputs.
### 4.2.1 RCC Register
The RCC register is a 32-bit register used to control the clock system. It includes several important fields:
- **CR:** Clock Control Register, used to control the selection of clock sources and the configuration of PLLs.
- **CFGR:** Clock Configuration Register, used to control the configuration of dividers.
- **CIR:** Clock Interrupt and Reset Register, used to control clock interrupts and resets.
- **APB1RSTR:** APB1 Bus Reset Register, used to reset peripherals on the APB1 bus.
- **APB2RSTR:** APB2 Bus Reset Register, used to reset peripherals on the APB2 bus.
### 4.2.2 Example of Clock Tree Configuration
Here is an example code snippet for configuring the clock tree:
```c
// Set clock source to HSE
RCC->CR |= RCC_CR_HSEON;
while((RCC->CR & RCC_CR_HSERDY) == 0);
// Set PLL multiplication factor to 8
RCC->CFGR |= RCC_CFGR_PLLMUL8;
// Set PLL division factor to 2
RCC->CFGR |= RCC_CFGR_PLLDIV2;
// Enable PLL
RCC->CR |= RCC_CR_PLLON;
while((RCC->CR & RCC_CR_PLLRDY) == 0);
// Set AHB division factor to 1
RCC->CFGR |= RCC_CFGR_HPRE_DIV1;
// Set APB1 division factor to 2
RCC->CFGR |= RCC_CFGR_PPRE1_DIV2;
// Set APB2 division factor to 4
RCC->CFGR |= RCC_CFGR_PPRE2_DIV4;
```
This code sets the clock source to HSE, PLL multiplication factor to 8, PLL division factor to 2, AHB division factor to 1, APB1 division factor to 2, and APB2 division factor to 4.
# 5. Clock System Applications
### 5.1 Clock Accuracy Analysis
Clock accuracy is an important metric for assessing clock system performance, directly impacting the accuracy of timers and counters within the system. Clock accuracy analysis mainly includes the analysis of clock source errors and division errors.
#### 5.1.1 Clock Source Errors
Clock source errors are mainly caused by:
- **Temperature Drift:** The frequency of a clock source changes with temperature variations, known as temperature drift. The temperature drift coefficient represents the relative rate of change of the clock source frequency with temperature.
- **Voltage Drift:** The frequency of a clock source also varies with changes in supply voltage, known as voltage drift. The voltage drift coefficient represents the relative rate of change of the clock source frequency with supply voltage.
- **Aging:** Over time, the frequency of a clock source gradually changes, known as aging. The aging coefficient represents the relative rate of change of the clock source frequency over time.
#### 5.1.2 Division Errors
Division errors are mainly caused by:
- **Division Ratio Error:** There is a certain error between the actual value and the design value of the division ratio in the divider, known as division ratio error. Division ratio error is mainly caused by process errors within the divider circuit and temperature drift.
- **Counting Error:** During the division process, there is a certain error between the counter's value and the actual number of divisions, known as counting error. Counting error is mainly caused by timing errors in the counter and noise interference.
### 5.2 Clock System Optimization
To enhance the accuracy and stability of the clock system, optimization is necessary. Clock system optimization mainly includes clock source selection, division configuration, and clock tree optimization.
#### 5.2.1 Clock Source Selection
Clock source selection mainly considers the following factors:
- **Accuracy:** Different clock sources have varying levels of accuracy, requiring the selection of an appropriate clock source based on the system's accuracy requirements.
- **Stability:** Clock source stability refers to how its frequency changes over time, necessitating the selection of clock sources with high stability.
- **Power Consumption:** Different clock sources have different power consumption levels, requiring the selection of clock sources that meet the system's power requirements.
#### 5.2.2 Division Configuration
Optimization of division configuration mainly considers the following factors:
- **Division Ratio:** The selection of the division ratio needs to be carefully considered based on the system's requirements for clock frequency and the accuracy of the clock source.
- **Division Error:** Division ratio error affects the accuracy of the clock system, requiring the selection of dividers with minimal division ratio errors.
- **Power Consumption:** The operating power consumption of dividers is related to the division ratio, requiring the selection of an appropriate division ratio based on the system's power requirements.
#### 5.2.3 Clock Tree Optimization
Optimization of the clock tree mainly considers the following factors:
- **Clock Path:** The length and topology of the clock path affect clock signal transmission delay and jitter. Optimizing the clock path can reduce delay and jitter.
- **Clock Isolation:** Different clock domains require isolation to prevent interference between clock signals.
- **Power Consumption:** The power consumption of the clock tree is related to the frequency of the clock signals and the transmission distance. Optimizing the clock tree can reduce power consumption.
0
0
相关推荐
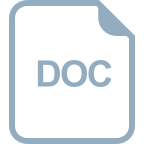
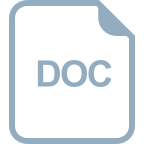
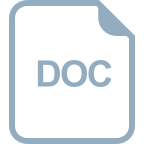
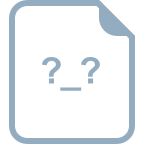
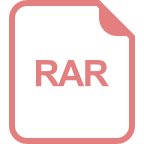
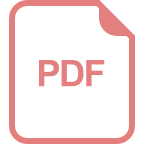
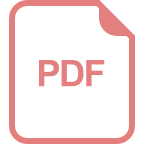
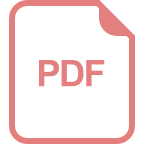
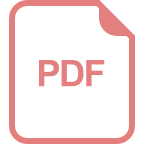