STM32 Microcontroller DMA Transmission Unveiled: In-depth Explanation of DMA Principles, Configuration, and Application for Efficient Data Transfer
发布时间: 2024-09-14 15:43:47 阅读量: 39 订阅数: 34 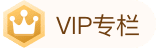
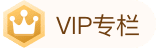
# 1. Overview of DMA Transfer
Direct Memory Access (DMA) is a hardware technique that enables peripherals to transfer data directly to and from memory without the intervention of the CPU. It optimizes system performance by reducing CPU overhead and enhancing the efficiency of data transfers.
The benefits of DMA transfers include:
- **Increased data transfer efficiency:** DMA can execute data transfers in parallel, freeing up CPU resources for other tasks.
- **Reduced CPU overhead:** The CPU does not participate in the data transfer process, thereby lowering CPU utilization.
- **Support for high-speed data transfers:** DMA can handle high-bandwidth data transfers, meeting the needs of real-time applications.
# 2. The Principle of DMA
### 2.1 Structure and Operation of DMA
DMA (Direct Memory Access) is a hardware module that allows peripherals to access system memory directly, without CPU intervention. It operates in the following manner:
- **DMA Controller:** The core component of DMA management, responsible for configuring and controlling data transfers. It includes registers for setting up and controlling transmissions.
- **DMA Channels:** Each DMA controller has multiple channels, each responsible for a specific peripheral or memory area.
- **DMA Request (DMA Request):** When a peripheral requires data transfer, it sends a DMA request to the DMA controller.
- **DMA Acknowledge (DMA Acknowledge):** If the DMA controller can process the request, it sends a DMA acknowledge to the peripheral, indicating that the transfer can commence.
- **DMA Transfer:** The DMA controller transfers data from the source address to the target address without CPU intervention.
### 2.2 DMA Transfer Modes and Types
DMA supports a variety of transfer modes and types to accommodate different application scenarios:
**Transfer Modes:**
- **Single Transfer:** The DMA controller executes one transfer and then stops.
- **Circular Transfer:** The DMA controller repeatedly transfers data until a specified number of transfers or conditions are met.
**Transfer Types:**
- **Memory-to-Memory Transfer:** DMA controller transfers data between two memory areas.
- **Peripheral-to-Memory Transfer:** DMA controller transfers data from a peripheral to memory.
- **Memory-to-Peripheral Transfer:** DMA controller transfers data from memory to a peripheral.
### 2.3 DMA Priority and Interrupt Mechanism
DMA controllers typically have configurable priorities, allowing different channels to be assigned different priorities. When multiple DMA requests occur simultaneously, the channel with higher priority will be processed first.
DMA controllers also support an interrupt mechanism; upon the completion of a transfer or the occurrence of an error, they generate an interrupt. This allows the CPU to handle DMA events without continuously polling the DMA status.
**Code Block:**
```c
// Configure DMA priority
HAL_DMA_SetPriority(DMA1_Channel1, DMA_PRIORITY_HIGH);
// Enable DMA interrupts
HAL_DMA_EnableIT(DMA1_Channel1);
// DMA transfer complete interrupt handler function
void DMA1_Channel1_IRQHandler(void)
{
// Handle DMA transfer complete event
}
```
**Logical Analysis:**
* The `HAL_DMA_SetPriority()` function is used to set the priority of the DMA channel.
* The `HAL_DMA_EnableIT()` function is used to enable DMA interrupts.
* The `DMA1_Channel1_IRQHandler()` function is a DMA transfer complete interrupt handler function, which handles the DMA transfer complete event.
# 3. DMA Configuration
### 3.1 Introduction to DMA Registers
The DMA controller of the STM32 microcontroller has a wealth of registers for configuring and controlling DMA transfers. The main registers include:
| Register | Function |
|---|---|
| DMA_CCRx | DMA channel configuration register, used to configure the transfer parameters of the channel |
| DMA_CNDTRx | DMA channel data transfer quantity register, used to set the amount of data to be transferred |
| DMA_CPARx | DMA channel peripheral address register, used to set the address of the peripheral |
| DMA_CMARx | DMA channel memory address register, used to set the address of memory |
| DMA_ISR | DMA interrupt status register, used to indicate the status of DMA interrupts |
| DMA_IFCR | DMA interrupt flag clear register, used to clear DMA interrupt flags |
### 3.2 DMA Channel Configuration
The DMA channel configuration register (DMA_CCRx) is used to configure the transfer parameters of the DMA channel, including:
- **DIR: Transfer Direction**
- 0: Memory to peripheral
- 1: Peripheral to memory
- **CIRC: Circular Mode**
- 0: Single transfer
- 1: Circular transfer
- **PINC: Peripheral Address Increment**
- 0: No increment
- 1: Increment
- **MINC: Memory Address Increment**
- 0: No increment
- 1: Increment
- **PL: Priority**
- 0-3: Priority from low to high
### 3.3 DMA Transfer Parameter Configuration
The DMA ch
0
0
相关推荐
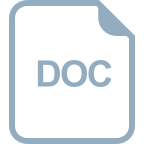
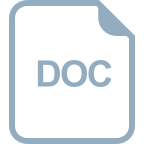
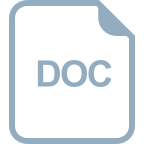
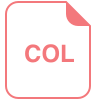
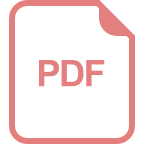
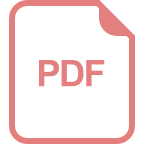
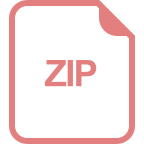
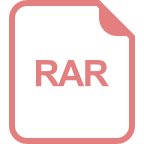
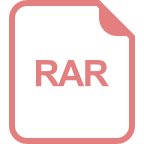