std::string replace
时间: 2023-09-10 12:06:13 浏览: 53
std::string replace是一个C++的字符串类std::string中的成员函数,用于替换字符串中的某个子串。它接受两个参数,第一个参数是要被替换的子串,第二个参数是替换后的新子串。该函数将返回一个新的std::string对象,其中包含替换完成后的字符串。
例如,假设有一个字符串str = "Hello, World!",要将其中的"World"替换为"Universe",可以使用replace函数:
```cpp
std::string str = "Hello, World!";
str.replace(str.find("World"), 5, "Universe");
```
在上述代码中,find函数用于查找子串"World"的起始位置,并返回其索引。然后,replace函数将从该起始位置开始的5个字符替换为"Universe"。因此,str将被修改为"Hello, Universe!"。
需要注意的是,如果要被替换的子串在原始字符串中出现了多次,replace函数只会替换第一次出现的子串。如果要替换所有出现的子串,可以使用循环或递归来实现。
希望这可以解答你的问题!如果还有其他问题,请随时提问。
相关问题
std::string replace 替换字符串
std::string的replace函数可以用于替换字符串中的子串。它的函数签名如下:
```cpp
std::string& replace(size_t pos, size_t count, const std::string& str);
```
其中,pos表示要替换的起始位置,count表示要替换的字符数,str表示用于替换的字符串。
replace函数会将原字符串中从pos位置开始的count个字符替换为str。如果str的长度小于count,则只会替换掉count个字符;如果str的长度大于count,则会替换掉count个字符,并在pos位置插入str中多出来的字符。
下面是一个示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "原始字符串:" << str << std::endl;
str.replace(7, 5, "C++");
std::cout << "替换后的字符串:" << str << std::endl;
return 0;
}
```
输出结果为:
```
原始字符串:Hello, world!
替换后的字符串:Hello, C++!
```
std::string.replace
std::string.replace是C++标准库中std::string类的一个成员函数,用于替换字符串中的一部分内容。它的函数原型如下:
```cpp
std::string& replace(size_t pos, size_t count, const std::string& str);
```
参数说明:
- pos:要替换的起始位置。
- count:要替换的字符数。
- str:用于替换的字符串。
该函数会将字符串中从pos位置开始的count个字符替换为str。替换后的字符串会被修改,并且返回一个对修改后的字符串的引用。
下面是一个使用std::string.replace的示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "Before replace: " << str << std::endl;
str.replace(7, 5, "C++");
std::cout << "After replace: " << str << std::endl;
return 0;
}
```
输出结果为:
```
Before replace: Hello, world!
After replace: Hello, C++!
```
在上面的示例中,我们将字符串中从位置7开始的5个字符替换为"C++",得到了替换后的字符串"Hello, C++!"。
相关推荐
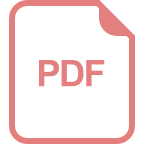
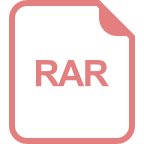
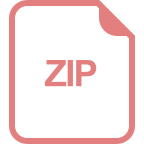












