std::string 字符替换
时间: 2024-03-11 09:42:38 浏览: 91
std::string是C++标准库中的一个类,用于处理字符串。在std::string中,可以使用replace()函数来进行字符替换操作。
replace()函数有多个重载版本,其中最常用的版本如下:
```cpp
std::string& replace(size_t pos, size_t count, const std::string& str);
```
这个版本的replace()函数将从指定位置pos开始,替换count个字符为str。替换后的结果会直接修改原字符串。
以下是一个示例代码,演示了如何使用replace()函数进行字符替换:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "原始字符串: " << str << std::endl;
str.replace(7, 5, "CSDN");
std::cout << "替换后的字符串: " << str << std::endl;
return 0;
}
```
输出结果为:
```
原始字符串: Hello, world!
替换后的字符串: Hello, CSDN!
```
相关问题
std::string替换字符串
在C++中,可以使用`std::string`类的`replace()`函数来替换字符串。该函数的原型为:
```
std::string& replace (size_t pos, size_t len, const std::string& str);
```
其中,`pos`表示要替换的子串在原字符串中的起始位置,`len`表示要替换的子串的长度,`str`表示用来替换的新字符串。
例如,如果要将字符串中的某一部分替换为另一个字符串,可以使用类似下面的代码:
```cpp
std::string str = "hello world";
std::string new_str = "there";
str.replace(6, 5, new_str);
```
这将把字符串中从位置6开始长度为5的子串(即"world")替换为新字符串"there",得到的结果为"hello there"。
std::string replace 替换字符串
std::string的replace函数可以用于替换字符串中的子串。它的函数签名如下:
```cpp
std::string& replace(size_t pos, size_t count, const std::string& str);
```
其中,pos表示要替换的起始位置,count表示要替换的字符数,str表示用于替换的字符串。
replace函数会将原字符串中从pos位置开始的count个字符替换为str。如果str的长度小于count,则只会替换掉count个字符;如果str的长度大于count,则会替换掉count个字符,并在pos位置插入str中多出来的字符。
下面是一个示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "原始字符串:" << str << std::endl;
str.replace(7, 5, "C++");
std::cout << "替换后的字符串:" << str << std::endl;
return 0;
}
```
输出结果为:
```
原始字符串:Hello, world!
替换后的字符串:Hello, C++!
```
阅读全文
相关推荐
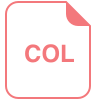












