如何在汇编语言中设计一个程序,接收用户输入两个五位数,将它们相加后并在屏幕上显示出计算结果?
时间: 2024-11-10 14:14:52 浏览: 32
在汇编语言中设计一个接收用户输入并显示计算结果的简单程序,你需要结合操作系统提供的功能和基本的输入/输出操作。下面是一个基于Linux环境下的汇编代码示例,使用x86体系结构,特别是使用GCC汇编器链接的裸机汇编代码,因为Linux提供了一些系统调用来获取用户输入和打印结果。
```assembly
section .data
prompt db '请输入第一个五位数:',0
prompt_len equ $ - prompt
number_format db '%d',0
separator db ' + ',0
result_prompt db '结果是:',0
result_prompt_len equ $ - result_prompt
max_length equ 5 * sizeof(db)
section .bss
input resb max_length ; 用户输入区域
result resb max_length ; 结果区域
section .text
extern printf, scanf, getchar, exit
get_input:
push ebp
mov ebp, esp
sub esp, max_length
mov eax, 4 ; sys_write for print prompt
mov ebx, 1 ; stdout
mov ecx, prompt ; pointer to prompt string
mov edx, prompt_len
int 0x80 ; call kernel
; read user input
mov eax, 3 ; sys_read for user input
mov ebx, 0 ; stdin
mov ecx, esp ; buffer for input
mov edx, max_length
int 0x80
; convert input to number and store in result
lea edi, [esp + max_length]
mov eax, [edi]
sub eax, '0' ; ASCII value of '0'
mov byte [result], al
mov eax, eax ; increment eax for next digit
loop_get_input:
call getchar
cmp al, '9' ; check if digit or end of input
jl end_input
sub al, '0' ; convert to decimal
mov byte [edi], al
inc edi ; move to next position
inc eax ; advance for next digit
jmp loop_get_input
end_input:
; calculate sum
mov ebx, esp ; point ebx to start of result
xor ecx, ecx ; clear ecx for counter
sum_loop:
add al, [esi] ; add current digit from input
add al, [ebx] ; add current digit from result
add byte [ebx], al
rol ebx, 1 ; shift left to make room for new digit
inc ecx ; increment count
cmp ecx, 5 ; check if we've added all digits
jle sum_loop
; display the result
mov eax, 4 ; sys_write for print result prompt
mov ebx, 1 ; stdout
mov ecx, result_prompt
mov edx, result_prompt_len
int 0x80 ; call kernel
mov eax, 4 ; sys_write for print result
mov ebx, 1 ; stdout
mov ecx, result ; pointer to result string
mov edx, eax ; use eax as length (which contains the result's size)
int 0x80 ; call kernel
; cleanup and exit
mov eax, 1 ; sys_exit
xor ebx, ebx ; return code 0
int 0x80 ; call kernel
end:
pop ebp
ret
```
请注意,这个示例假设用户输入的是正整数且不超过五位。实际应用中,你可能需要增加错误检查和处理部分,以及改进数据验证。
阅读全文
相关推荐
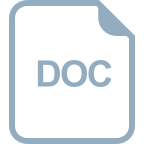
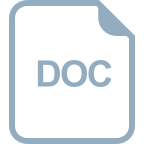
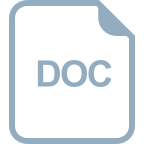
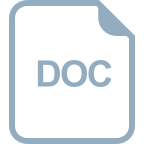
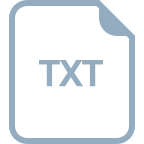
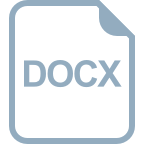
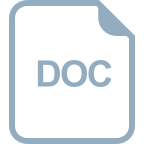
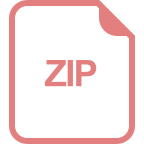
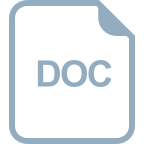
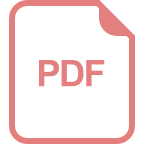
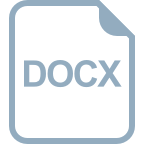
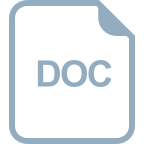
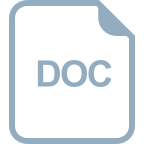
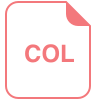
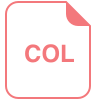
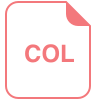
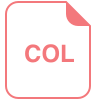
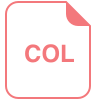
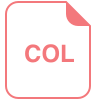