编写代码,实现一个基于面向对象思想的、具有添加、删除、修改、查找联系人信息,以及查看所有联系人的信息功能的通讯录程序。
时间: 2024-12-21 08:29:42 浏览: 0
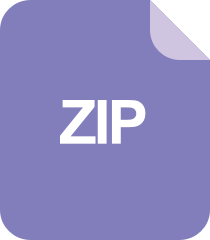
java班级管理系统(java+面向对象+源码)

当然,我们可以创建一个简单的通讯录程序来演示面向对象的概念。在这个例子中,我们假设会有一个名为`ContactBook`的类作为核心容器,它包含一个列表`contacts`来存储`Contact`对象。
```python
class Contact:
def __init__(self, id, name, phone_number):
self.id = id
self.name = name
self.phone_number = phone_number
class ContactBook:
def __init__(self):
self.contacts = []
# 添加联系人
def add_contact(self, contact):
self.contacts.append(contact)
# 删除联系人
def delete_contact(self, id):
for contact in self.contacts:
if contact.id == id:
self.contacts.remove(contact)
break
# 修改联系人信息
def update_contact(self, id, new_name=None, new_phone_number=None):
for contact in self.contacts:
if contact.id == id:
if new_name:
contact.name = new_name
if new_phone_number:
contact.phone_number = new_phone_number
return
print("联系人未找到")
# 查找联系人
def find_contact(self, id):
for contact in self.contacts:
if contact.id == id:
return contact
return None
# 查看所有联系人
def view_all_contacts(self):
return self.contacts
# 示例使用
contact_book = ContactBook()
contact1 = Contact(1, "张三", "1234567890")
contact_book.add_contact(contact1)
# 打印所有联系人
print("初始联系人:")
for contact in contact_book.view_all_contacts():
print(f"ID: {contact.id}, Name: {contact.name}, Phone: {contact.phone_number}")
# 更新和查找
contact_book.update_contact(1, new_name="李四")
print("\n更新后的联系人:")
for contact in contact_book.view_all_contacts():
print(f"ID: {contact.id}, Name: {contact.name}, Phone: {contact.phone_number}")
found_contact = contact_book.find_contact(1)
if found_contact:
print(f"找到了联系人,Name: {found_contact.name}, Phone: {found_contact.phone_number}")
else:
print("没有找到联系人")
# 删除联系人
contact_book.delete_contact(1)
print("\n删除联系人后:")
for contact in contact_book.view_all_contacts():
print(f"ID: {contact.id}, Name: {contact.name}, Phone: {contact.phone_number}")
```
阅读全文
相关推荐
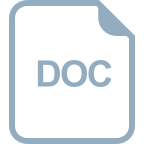
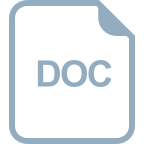
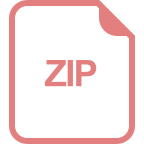
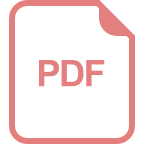
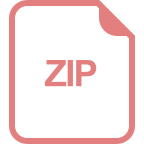
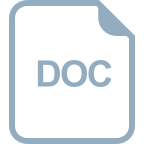
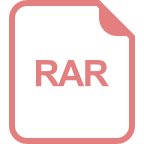
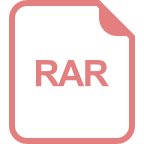
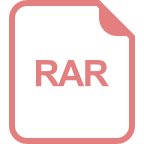
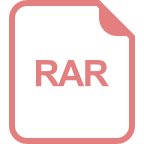
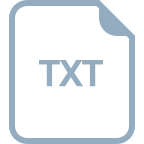
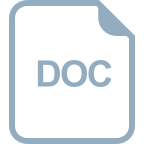
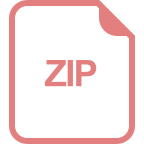
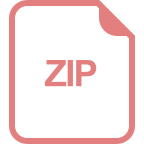
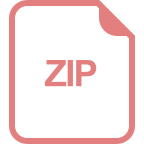
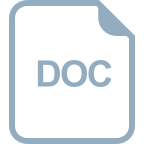
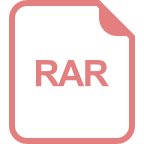
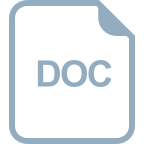