c语言编写猜数游戏程序
时间: 2024-06-13 21:06:40 浏览: 223
以下是一个简单的C语言猜数字游戏程序,程序会随机生成一个1到100之间的数字,玩家需要在提示下猜出这个数字:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int guess, num, count = 0;
srand(time(NULL));
num = rand() % 100 + 1;
printf("欢迎来到猜数字游戏!\n");
while (1) {
printf("请输入您猜的数字:");
scanf("%d", &guess);
count++;
if (guess > num) {
printf("您猜的数字偏大!\n");
} else if (guess < num) {
printf("您猜的数字偏小!\n");
} else {
printf("恭喜您猜对了!您一共猜了%d次。\n", count);
break;
}
}
return 0;
}
```
相关问题
用C语言编写猜数程序,程序中预先给定某个整数(例如100),用户从键盘反复输入整数进行猜数,每次输入数未猜中时,程序提示输入数过大或过小。猜中时是示猜的次数,最多允许猜3次。
以下是一个简单的猜数程序示例:
```c
#include <stdio.h>
int main()
{
const int answer = 100; //预设答案
int guess, count = 0; //猜测的数和猜测次数
printf("Guess the number (0~999) in 3 tries:\n");
do {
printf("Guess #%d: ", count+1);
scanf("%d", &guess);
if (guess < answer) {
printf("Too low!\n");
} else if (guess > answer) {
printf("Too high!\n");
}
count++;
} while (guess != answer && count < 3);
if (guess == answer) {
printf("You got it in %d tries!\n", count);
} else {
printf("Sorry, you didn't guess the number. It was %d.\n", answer);
}
return 0;
}
```
程序中用 `const int answer = 100;` 预先设定了答案为100。然后用一个 `do-while` 循环来反复提示用户输入猜测的数,并根据输入的数和答案进行比较,输出相应的提示信息。如果猜对了,输出猜测的次数;如果猜错了三次,输出正确答案。注意猜测次数的计数要在循环中进行。
C语言编程。编写猜数游戏程序,计算机自动随机生成一个100以内的整数,客户从键盘输入一个整数,系统提示猜大了、猜小了还是猜对了,重复输入直到猜对为止
在C语言中,我们可以创建一个简单的猜数游戏程序,通过`rand()`和`srand()`函数生成随机数,然后让用户通过循环不断猜测直到猜对。以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 引入time头文件以获取当前时间作为随机数种子
// 游戏主函数
int guessTheNumber() {
srand(time(0)); // 设置随机数种子,保证每次运行程序时都会得到不同的随机数
int secretNum = rand() % 101 + 1; // 生成1到100之间的随机数
while (true) {
int userGuess;
printf("I'm thinking of a number between 1 and 100. Guess the number:\n");
scanf("%d", &userGuess);
if (userGuess > secretNum) {
printf("Too high! Guess again.\n");
} else if (userGuess < secretNum) {
printf("Too low! Guess again.\n");
} else {
printf("Congratulations! You guessed the number %d correctly!\n", secretNum);
break;
}
}
return 0;
}
int main() {
guessTheNumber(); // 调用游戏主函数
return 0;
}
```
当用户运行此程序时,他们将看到提示并尝试猜测正确的数字。如果猜得太大或太小,程序会给出相应的提示。
阅读全文
相关推荐
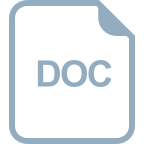
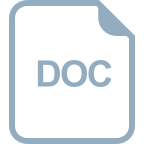
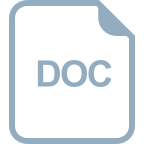
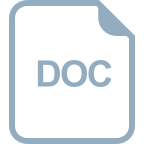
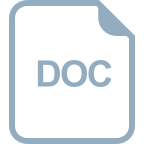
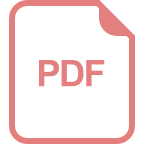
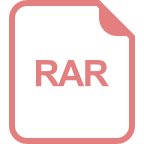
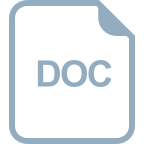
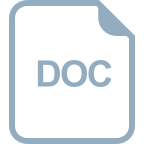
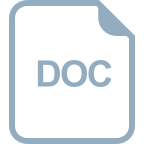






