c++中输出到文件中,怎么在原有文件换行输入
时间: 2024-11-15 17:33:06 浏览: 19
在 C++ 中,要将内容追加到已有文件并在每一行结尾添加换行符("\n"),你可以使用 `ofstream` 类。下面是一个简单的示例:
```cpp
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("example.txt", std::ios_base::app); // "app" 参数表示追加模式
if (outputFile.is_open()) {
// 想要输入的数据
std::string line1 = "这是第一行";
std::string line2 = "这是第二行";
// 写入文件,并在每行末尾添加换行符
outputFile << line1 << "\n";
outputFile << line2 << "\n";
// 输出成功信息
std::cout << "数据已成功写入文件。\n";
// 关闭文件
outputFile.close();
} else {
std::cerr << "无法打开文件 for writing.\n";
}
return 0;
}
```
在这个例子中,`std::ios_base::app` 模式告诉流在文件末尾追加而不是覆盖原有内容。如果文件不存在,它会自动创建。
相关问题
定义文件输入流对象ifstream fi(“fi.txt”),在文件中通过键盘输入“hello world”并保存,然后定义文件输出流对象ofstream fo(“fo.txt”),将fi中的文字拷贝到fo文件中的C++代码
在C++中,操作文件通常涉及`fstream`库,包括`ifstream`用于读取和`ofstream`用于写入。以下是实现你所描述功能的一个简单示例:
```cpp
#include <fstream>
#include <iostream>
int main() {
std::ifstream fi("fi.txt");
if (!fi) { // 检查文件是否成功打开
std::cerr << "Failed to open input file 'fi.txt'" << std::endl;
return 1;
}
std::string user_input;
std::cout << "Please enter some text: ";
std::getline(std::cin, user_input); // 从用户获取输入
// 尝试写入"fi.txt"
if (!fi) {
std::cerr << "Failed to read from 'fi.txt'" << std::endl;
return 1;
}
fi.seekg(0, std::ios::end); // 移动到文件末尾
fi.close(); // 关闭输入流
std::ofstream fo("fo.txt");
if (!fo) {
std::cerr << "Failed to create output file 'fo.txt'" << std::endl;
return 1;
}
// 写入用户输入内容到fo.txt
fo.write(user_input.c_str(), user_input.size());
fi.open("fi.txt"); // 为了覆盖原有内容,再次打开输入流
fi << user_input << std::endl; // 写入用户输入并添加换行
fi.close();
fo.close();
return 0;
}
```
c++清空本地某文件的一行内容再写入新的内容
可以使用C++的文件流来实现清空本地某文件的一行内容再写入新的内容。具体步骤如下:
1. 打开文件并创建输入输出流:
```cpp
#include <fstream>
using namespace std;
string filename = "test.txt";
fstream file(filename, ios::in | ios::out | ios::app);
```
其中 `ios::in` 表示以输入模式打开文件, `ios::out` 表示以输出模式打开文件, `ios::app` 表示以追加模式打开文件。
2. 定位到需要修改的行:
```cpp
string line;
int lineNumber = 3; // 需要修改的行号
while (getline(file, line)) {
lineNumber--;
if (lineNumber == 0) {
break;
}
}
```
这里假设需要修改第三行的内容,通过循环逐行读取文件内容,当读到第三行时跳出循环。
3. 清空该行内容:
```cpp
file.seekp(-line.length(), ios::cur);
file << string(line.length(), ' ');
file.seekp(-line.length(), ios::cur);
```
`seekp()` 函数用于定位输出流的位置,第一个参数表示偏移量,第二个参数表示偏移起始位置,`ios::cur` 表示相对于当前位置偏移。这里将输出流定位到当前行的开头,然后输出与该行长度相同的空格覆盖原有内容,再将输出流定位到该行开头。
4. 写入新的内容:
```cpp
string newContent = "This is a new line.";
file << newContent << endl;
```
将新的内容写入文件并换行。
5. 关闭文件:
```cpp
file.close();
```
完整代码如下:
```cpp
#include <fstream>
using namespace std;
int main() {
string filename = "test.txt";
fstream file(filename, ios::in | ios::out | ios::app);
string line;
int lineNumber = 3;
while (getline(file, line)) {
lineNumber--;
if (lineNumber == 0) {
break;
}
}
file.seekp(-line.length(), ios::cur);
file << string(line.length(), ' ');
file.seekp(-line.length(), ios::cur);
string newContent = "This is a new line.";
file << newContent << endl;
file.close();
return 0;
}
```
注意:为了方便起见,这里假设文件中至少有三行内容。如果文件行数不足三行,代码可能会出现错误。
阅读全文
相关推荐
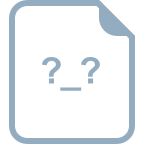
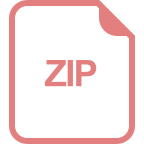
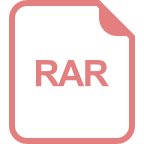
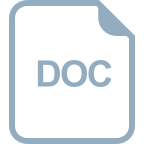
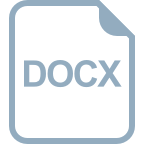
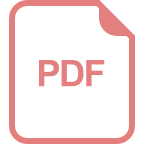
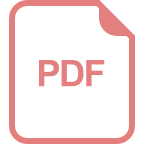
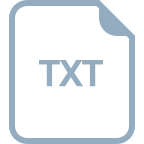
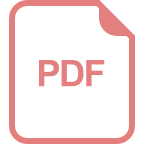
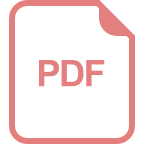
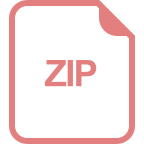
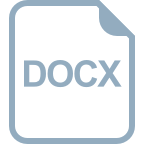
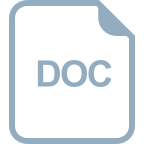
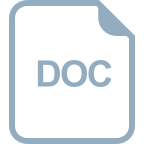
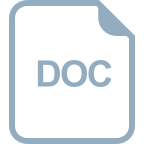
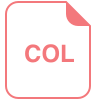
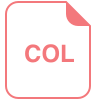
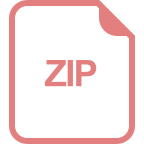