java swing画图板
时间: 2023-11-20 10:56:07 浏览: 103
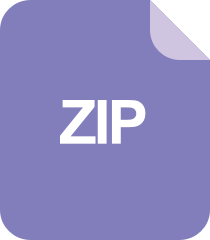
java swing 画图板

Java Swing是Java平台的一部分,是一组GUI类库,可以用于创建桌面应用程序。下面是一个简单的Java Swing画图板的实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class DrawingBoard extends JFrame implements ActionListener, MouseListener, MouseMotionListener {
private int x, y, x1, y1;
private JButton clearButton, redButton, greenButton, blueButton;
private JPanel buttonPanel, drawingPanel;
private Graphics g;
public DrawingBoard() {
super("Drawing Board");
setSize(500, 500);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
buttonPanel = new JPanel();
clearButton = new JButton("Clear");
redButton = new JButton("Red");
greenButton = new JButton("Green");
blueButton = new JButton("Blue");
buttonPanel.add(clearButton);
buttonPanel.add(redButton);
buttonPanel.add(greenButton);
buttonPanel.add(blueButton);
drawingPanel = new JPanel();
drawingPanel.setBackground(Color.WHITE);
drawingPanel.addMouseListener(this);
drawingPanel.addMouseMotionListener(this);
Container contentPane = getContentPane();
contentPane.add(buttonPanel, BorderLayout.NORTH);
contentPane.add(drawingPanel, BorderLayout.CENTER);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
g.setColor(Color.WHITE);
g.fillRect(0, 0, drawingPanel.getWidth(), drawingPanel.getHeight());
} else if (e.getSource() == redButton) {
g.setColor(Color.RED);
} else if (e.getSource() == greenButton) {
g.setColor(Color.GREEN);
} else if (e.getSource() == blueButton) {
g.setColor(Color.BLUE);
}
}
public void mousePressed(MouseEvent e) {
x = e.getX();
y = e.getY();
}
public void mouseDragged(MouseEvent e) {
x1 = e.getX();
y1 = e.getY();
g.drawLine(x, y, x1, y1);
x = x1;
y = y1;
}
public void mouseClicked(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseMoved(MouseEvent e) {}
public static void main(String[] args) {
new DrawingBoard();
}
}
```
阅读全文
相关推荐
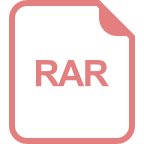
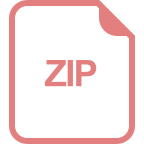
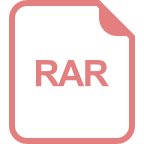
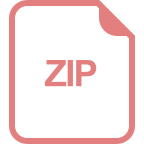
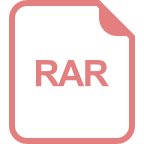
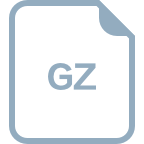
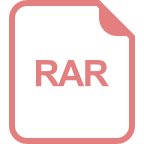
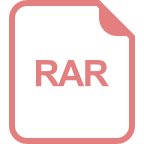
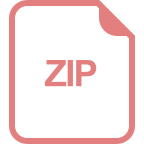
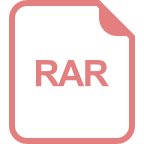
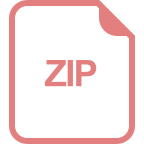
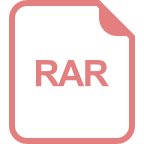
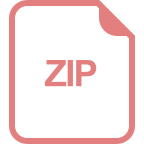
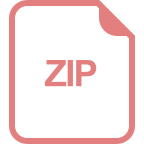
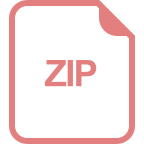
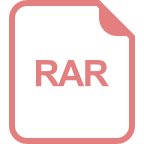