在c++中请解释一下以下格式<dog::type,int>
时间: 2024-09-29 09:09:58 浏览: 20
在 C++ 中,`<dog::type, int>` 这样的格式通常用于模板元编程或者是类型别名的定义。这里有两个部分组成:
1. `dog::type`: 这是一个名称限定,`dog` 可能是一个命名空间(namespace)或是一个类(class)。`type` 是在这个命名空间或类下的某个类型。通过 `<...>` 的形式,程序员明确指定了要在后续代码中使用这个类型的名称。
2. `int`: 第二个元素 `int` 表示另一个类型,这里是基本类型 `int`。
如果这是一个模板,可能是这样使用的:
```cpp
template <typename T>
struct AnimalType {
using TypePair = std::pair<T, int>;
};
```
这里 `AnimalType` 模板接受一个类型参数 `T`,然后定义了一个内部类型别名 `TypePair`,它将 `T` 类型和 `int` 类型组合成一对。
或者如果是类型别名,可能像这样:
```cpp
namespace dog {
struct DogType;
}
using DogAndInt = std::tuple<dog::DogType, int>;
```
这里的 `DogAndInt` 是一个类型别名,它表示 `dog` 命名空间下的 `DogType` 类型和 `int` 类型组成的 `std::tuple`。
相关问题
The following is an attempt to create a class to represent information about pets: class Pet { public: Pet(); void printDescription(); string name; int type; bool neuterSpayed; bool talks; }; Pet::Pet() : type(0), neuterSpayed(false), talks(false) { } void Pet::printDescription() { switch (type) { case 0: cout << "Dog named " << name << endl; cout << "Neuter/Spayed: " << neuterSpayed; break; case 1: cout << "Cat named " << name << endl; cout << "Neuter/Spayed: " << neuterSpayed; break; case 2: cout << "Bird named " << name << endl; cout << "Talks: " << talks << endl; break; } cout << endl; } Rewrite this code using inheritance. You should have a Pet class with subclasses for Dog, Cat, and Bird. Variables should be associated with the appropriate classes, defined as private when appropriate, and have appropriate functions to access the variables. Rewrite the printDescription function as a virtual function. There should no longer be the need for a switch statement or a type variable. Write a main function that creates a vector or array of pets that includes at least one bird, one dog, and one cat, and then loops through and outputs a description of each one.
Here's the updated code:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Pet {
public:
Pet(string name) : m_name(name) {}
virtual void printDescription() = 0; // make it pure virtual to make Pet an abstract class
protected:
string m_name;
};
class Dog : public Pet {
public:
Dog(string name, bool neutered) : Pet(name), m_neutered(neutered) {}
void printDescription() override {
cout << "Dog named " << m_name << endl;
cout << "Neuter/Spayed: " << m_neutered << endl;
}
private:
bool m_neutered;
};
class Cat : public Pet {
public:
Cat(string name, bool neutered) : Pet(name), m_neutered(neutered) {}
void printDescription() override {
cout << "Cat named " << m_name << endl;
cout << "Neuter/Spayed: " << m_neutered << endl;
}
private:
bool m_neutered;
};
class Bird : public Pet {
public:
Bird(string name, bool talks) : Pet(name), m_talks(talks) {}
void printDescription() override {
cout << "Bird named " << m_name << endl;
cout << "Talks: " << m_talks << endl;
}
private:
bool m_talks;
};
int main() {
vector<Pet*> pets;
pets.push_back(new Dog("Fido", true));
pets.push_back(new Cat("Whiskers", false));
pets.push_back(new Bird("Polly", true));
for (Pet* pet : pets) {
pet->printDescription();
}
// free memory
for (Pet* pet : pets) {
delete pet;
}
return 0;
}
```
In this updated code, we've used inheritance to create subclasses for Dog, Cat, and Bird. Each subclass has its own private variables `m_neutered` and `m_talks`, respectively. The `printDescription` function is now a virtual function in the `Pet` class, which means that each subclass can override it with its own implementation.
In the main function, we create a vector of `Pet` pointers and add instances of each subclass to it. We then loop through the vector and call the `printDescription` function on each `Pet` object, which automatically calls the appropriate subclass implementation of the function. Finally, we free the memory allocated for each `Pet` object using `delete`.
定义基类 Animal, 包含保护数据成员 name 和纯虛函数talk(),定义猫类 cat,继承 Animal 类,实现 talk()方法,输出为:cout<<name<< “MiaoMiao” <<endl;定义狗类 Dog;继承Animal 类,实现talk()方法,输出为: cout<<name<< "WangWang" <<endl. 输入描述:有键盘输入动物个数和每种动物的信息,包括动物关型 (其中C 表示猫类,D表示正方形类)及其 相应名称。 猫的输入格式为:C名字 狗的输入格式为:D名字 输出描述:通过 Animal 类的对象指针调用虚函数 talk(),依次输出每种动物的叫的动作。 猫的信息输出格式为: Cat 的名字叫的动作 狗的信息输出格式为: Dog 的名字叫的动作
好的,以下是 C++ 代码实现:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Animal {
protected:
string name;
public:
virtual void talk() = 0;
};
class Cat : public Animal {
public:
Cat(string n) {
name = n;
}
void talk() {
cout << "Cat " << name << " MiaoMiao" << endl;
}
};
class Dog : public Animal {
public:
Dog(string n) {
name = n;
}
void talk() {
cout << "Dog " << name << " WangWang" << endl;
}
};
int main() {
int n;
cin >> n;
vector<Animal*> animals;
for (int i = 0; i < n; i++) {
char type;
string name;
cin >> type >> name;
if (type == 'C') {
animals.push_back(new Cat(name));
} else if (type == 'D') {
animals.push_back(new Dog(name));
}
}
for (auto animal : animals) {
animal->talk();
}
return 0;
}
```
输入格式为:
```
动物个数
C/D 名字
C/D 名字
...
```
例如:
```
3
C Tom
D Jerry
C Kitty
```
输出结果为:
```
Cat Tom MiaoMiao
Dog Jerry WangWang
Cat Kitty MiaoMiao
```
阅读全文
相关推荐
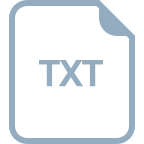
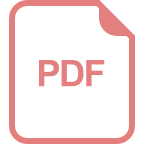
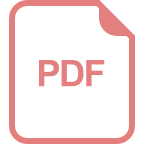
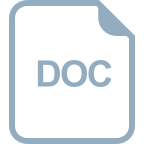
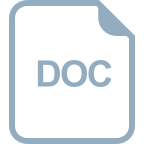
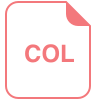
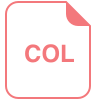
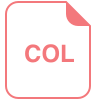
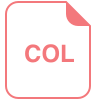
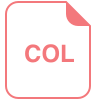
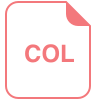
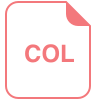
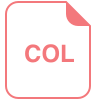
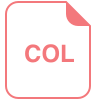
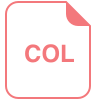
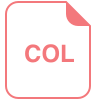