用C++实现一个判断所给中根序列与后根序列是否能组成同一颗树的算法
时间: 2024-11-04 19:15:28 浏览: 11
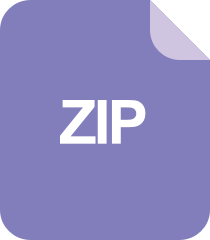
动态规划算法的c++实现 能实现两序列的比对
在C++中,判断一个给定的中根序列(即二叉树的前序遍历结果)和后根序列(即二叉树的后序遍历结果),能否构成同一棵树,可以利用递归的方法来解决。这里需要两个辅助函数:前序遍历和后序遍历。
首先,我们需要了解中根序列和后根序列的特点:
- 中根序列:先访问根节点,然后遍历左子树,最后遍历右子树。
- 后根序列:先访问左子树,然后遍历右子树,最后访问根节点。
以下是一个简单的示例算法:
```cpp
#include <vector>
using namespace std;
// 辅助函数:将前序遍历转化为后序遍历的结果
vector<int> buildPostorder(vector<int>& preorder) {
stack<int> s;
int inOrderIndex = 0;
vector<int> postorder;
for (int num : preorder) {
if (num == 0) {
while (!s.empty() && s.top() != inOrderIndex) {
postorder.push_back(s.top());
s.pop();
inOrderIndex++;
}
s.pop(); // 出栈当前的前序节点
} else {
s.push(num);
}
}
while (!s.empty()) {
postorder.push_back(s.top());
s.pop();
}
return postorder;
}
// 主函数:判断中根序列和后根序列是否对应同一棵树
bool isSameTree(vector<int>& inorder, vector<int>& postorder) {
if (inorder.empty() || postorder.empty())
return inorder.empty() && postorder.empty();
return inorder[0] == postorder.back() &&
isSameTree(inorder.substr(1), postorder.substr(0, postorder.size() - 1));
}
阅读全文
相关推荐
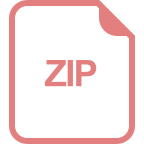
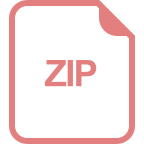


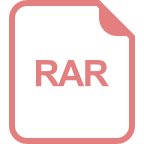
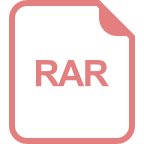
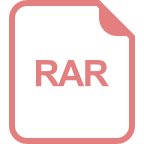
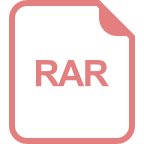
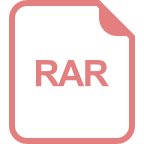
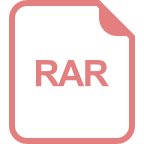
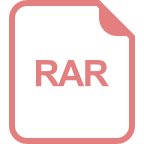
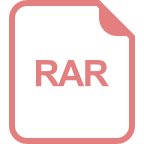
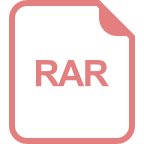
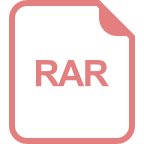
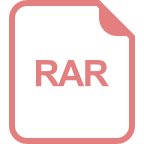
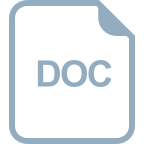
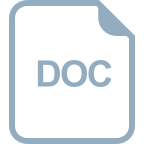