python接收一个列表,找出其中数量最多的元素,如果元素数量相同,选择列表中靠前的
时间: 2023-09-07 18:03:53 浏览: 83
要找出列表中数量最多的元素,可以使用Python的collections模块中的Counter类来实现。
首先,我们可以通过Counter类的most_common()方法将列表中的每个元素及其出现的次数计算出来,并按照出现次数从高到低的顺序排列。然后,我们可以取最多次数的元素即可。
示例代码如下:
```python
from collections import Counter
def find_most_common(lst):
counter = Counter(lst)
most_common = counter.most_common()
return most_common[0][0]
# 测试
lst = [1, 2, 3, 1, 2, 3, 4, 4, 4]
most_common_element = find_most_common(lst)
print("列表中数量最多的元素是:", most_common_element)
```
以上代码运行结果为:列表中数量最多的元素是: 4
在这个例子中,列表中的元素1出现2次,元素2出现2次,元素3出现2次,元素4出现3次,由于元素4的出现次数最多,所以输出结果为4。
如果列表中存在多个出现次数相同的元素,选择靠前的元素,可以稍作修改。修改后的代码如下:
```python
from collections import Counter
def find_most_common(lst):
counter = Counter(lst)
most_common = counter.most_common()
common_elements = [x[0] for x in most_common if x[1] == most_common[0][1]]
return common_elements[0]
# 测试
lst = [1, 2, 3, 1, 2, 3]
most_common_element = find_most_common(lst)
print("列表中数量最多的元素是:", most_common_element)
```
以上代码运行结果为:列表中数量最多的元素是: 1
在这个例子中,元素1和元素2都出现了2次,由于元素1在列表中靠前,所以输出结果为1。
相关推荐
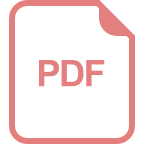
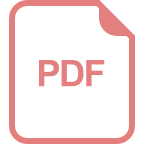
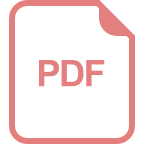














