Python植物大战僵尸种植植物代码
时间: 2024-08-11 13:01:14 浏览: 102
在Python中模拟《植物大战僵尸》游戏中的植物种植通常会涉及到一些基本的游戏逻辑,包括创建植物对象、设置生长位置等。这里是一个简单的示例,假设我们有一个基础的Plant类和几个具体的植物子类,如PeaShooter (豌豆射手) 和Sunflower (向日葵):
```python
class Plant:
def __init__(self, x, y):
self.x = x
self.y = y
# 其他初始化属性...
def plant(self):
print(f"Planting {type(self).__name__} at ({self.x}, {self.y})")
class PeaShooter(Plant):
def __init__(self, x, y):
super().__init__(x, y)
# 豌豆射手特定属性...
class Sunflower(Plant):
def __init__(self, x, y):
super().__init__(x, y)
# 向日葵特定属性...
# 示例使用:
def place_plant(row, column, plant_type):
if isinstance(plant_type, PeaShooter):
shooter = PeaShooter(column, row)
shooter.plant()
elif isinstance(plant_type, Sunflower):
sunflower = Sunflower(column, row)
sunflower.plant()
else:
print("Invalid plant type.")
# 游戏主循环
for _ in range(num_rows):
for _ in range(num_columns):
place_plant(_, _, PeaShooter) # 只放置豌豆射手作为例子
```
在这个例子中,`place_plant`函数接受行(row)、列(column)和植物类型(plant_type),然后根据类型实例化并种植植物。你可以根据需要添加更多种类的植物和相应的功能。
阅读全文
相关推荐
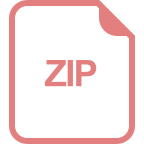
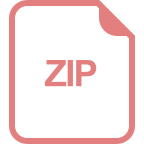
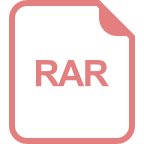
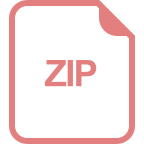
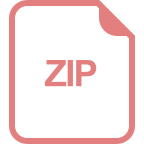
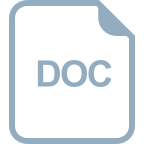
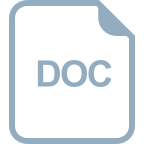








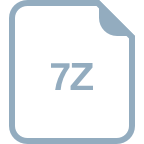

