将当前shell脚本承载业务使用python实现出来 cp /etc/pam.d/su /etc/pam.d/su.bak cp /etc/pam.d/password-auth-ac /etc/pam.d/password-auth-ac.bak cp /etc/pam.d/system-auth /etc/pam.d/system-auth.bak cp /etc/login.defs /etc/login.defs.bak cp /etc/ssh/sshd_config /etc/ssh/sshd_config.bak cp /etc/vsftpd/vsftpd.conf /etc/vsftpd/vsftpd.conf.bak cp /etc/profile /etc/profile.bak cp /etc/motd /etc/motd.bak
时间: 2023-07-14 17:07:58 浏览: 150
以下是将当前shell脚本承载业务使用Python实现的代码示例:
```python
import shutil
files_to_backup = [
'/etc/pam.d/su',
'/etc/pam.d/password-auth-ac',
'/etc/pam.d/system-auth',
'/etc/login.defs',
'/etc/ssh/sshd_config',
'/etc/vsftpd/vsftpd.conf',
'/etc/profile',
'/etc/motd'
]
for file_path in files_to_backup:
backup_path = file_path + '.bak'
shutil.copy(file_path, backup_path)
```
上述代码使用了Python的`shutil`模块中的`copy`函数来实现文件的备份。将需要备份的文件路径存储在`files_to_backup`列表中,然后遍历列表,对每个文件执行备份操作,将原文件复制到以`.bak`为后缀的备份文件中。
请确保你的Python环境中已经安装了`shutil`模块,并将需要备份的文件路径正确添加到`files_to_backup`列表中。运行代码后,文件备份操作将会被执行。
相关问题
python2 查看是否存在下面的文件并备份 cp /etc/pam.d/su /etc/pam.d/su.bak cp /etc/pam.d/password-auth-ac /etc/pam.d/password-auth-ac.bak cp /etc/pam.d/system-auth /etc/pam.d/system-auth.bak cp /etc/login.defs /etc/login.defs.bak cp /etc/ssh/sshd_config /etc/ssh/sshd_config.bak cp /etc/vsftpd/vsftpd.conf /etc/vsftpd/vsftpd.conf.bak cp /etc/profile /etc/profile.bak cp /etc/motd /etc/motd.bak
以下是将这些命令转换为Python代码的示例:
```python
import subprocess
files_to_backup = [
"/etc/pam.d/su",
"/etc/pam.d/password-auth-ac",
"/etc/pam.d/system-auth",
"/etc/login.defs",
"/etc/ssh/sshd_config",
"/etc/vsftpd/vsftpd.conf",
"/etc/profile",
"/etc/motd"
]
for file_path in files_to_backup:
backup_file_path = file_path + ".bak"
subprocess.call(["cp", file_path, backup_file_path])
print("Backup completed.")
```
上述代码使用循环来遍历要备份的文件路径列表。对于每个文件,它使用`cp`命令将原文件复制为具有`.bak`扩展名的备份文件。
请确保你具有足够的权限来执行这些操作,并将需要备份的文件路径添加到`files_to_backup`列表中。运行代码后,文件将被备份,并打印出`Backup completed.`。
python2批量在文件同文件夹下备份为[文件名]+[.bak] ``` /etc/pam.d/su /etc/pam.d/password-auth-ac /etc/pam.d/system-auth /etc/login.defs /etc/ssh/sshd_config /etc/vsftpd/vsftpd.conf /etc/profile /etc/motd ```
以下是使用Python 2批量备份文件的示例代码:
```python
import os
# 要备份的文件列表
files = [
"/etc/pam.d/su",
"/etc/pam.d/password-auth-ac",
"/etc/pam.d/system-auth",
"/etc/login.defs",
"/etc/ssh/sshd_config",
"/etc/vsftpd/vsftpd.conf",
"/etc/profile",
"/etc/motd"
]
# 遍历文件列表
for file_path in files:
# 获取文件名和文件所在的目录
file_dir, file_name = os.path.split(file_path)
# 构建备份文件名
backup_file_name = file_name + ".bak"
# 构建备份文件的完整路径
backup_file_path = os.path.join(file_dir, backup_file_name)
# 备份文件
os.rename(file_path, backup_file_path)
print("已备份文件:" + file_path + " -> " + backup_file_path)
```
请注意,这是使用Python 2编写的代码,对应的Python 3代码可能会有一些差异。
阅读全文
相关推荐
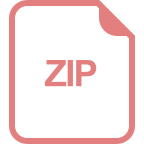
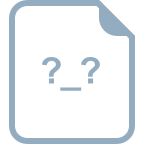
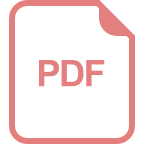







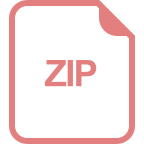
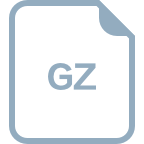
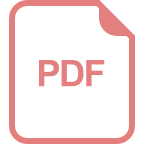
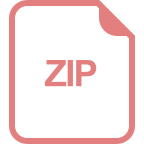