python list计算出现最多的数字
时间: 2024-12-30 11:38:48 浏览: 34
在Python中,如果你有一个列表并且想要找出其中出现次数最多的数字,你可以使用collections模dule中的Counter类来统计每个元素的出现次数,然后找到出现次数最多的元素。以下是一个简单的步骤:
```python
from collections import Counter
def most_common_number(lst):
# 使用Counter计算每个元素的频率
frequency = Counter(lst)
# 找到最常见的元素(如果有多个相同的最频繁值,则返回一个迭代器)
most_common = frequency.most_common(1)
# 如果列表不为空,返回最常见的元素及其频率,否则返回None或空字典
if lst:
return most_common if most_common else None
else:
return None
# 示例用法
numbers_list = [1, 2, 3, 2, 2, 4, 5, 2]
most_common = most_common_number(numbers_list)
print(f"出现次数最多的数字是 {most_common},出现了 {most_common}次")
```
运行上述代码后,它会输出列表中最常出现的数字以及它的出现次数。
相关问题
python如何返回矩阵中出现最多的数
在Python中,可以使用numpy库来处理矩阵(数组),并结合一些统计方法来找出出现次数最多的元素。以下是一个简单的步骤:
1. 首先,导入所需的numpy库:
```python
import numpy as np
```
2. 定义一个函数,接受一个二维数组(矩阵)作为输入:
```python
def most_frequent(matrix):
```
3. 将矩阵转换为一维数组以便于计数:
```python
flat_list = matrix.flatten()
```
4. 使用numpy的`mode()`函数计算出现频率最高的元素及其出现次数:
```python
mode_result = np.bincount(flat_list).argmax()
most_common_num = flat_list[mode_result]
```
5. 返回最常出现的数字:
```python
return most_common_num
```
完整代码示例:
```python
def most_frequent(matrix):
import numpy as np
flat_list = matrix.flatten()
mode_result = np.bincount(flat_list).argmax()
most_common_num = flat_list[mode_result]
return most_common_num
# 测试用例
matrix = np.array([[1, 2, 2], [2, 3, 2], [3, 4, 2]])
print(most_frequent(matrix)) # 输出最常见的数字
用Python计算列表中数字的平均值
可以使用以下代码计算列表中数字的平均值:
```
my_list = [1, 2, 3, 4, 5]
average = sum(my_list) / len(my_list)
print(average)
```
输出结果为:
```
3.0
```
其中,`sum(my_list)`计算列表中所有数字的总和,`len(my_list)`计算列表中数字的个数,然后将总和除以个数得到平均值。
阅读全文
相关推荐







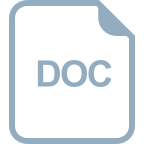








