javaspringboot,在impl和service层,control层,mapper层,xml文件中写出,更新相同产品名称product_id字段下的feeUnit,speedUnit,order_attention,cover_img_path字段的信息,使得这些信息相同
时间: 2024-04-10 17:33:29 浏览: 24
在Spring Boot中,你可以按照如下的方式在Impl、Service、Controller、Mapper和XML文件中编写代码来更新相同产品名称(product_id字段)下的feeUnit、speedUnit、order_attention和cover_img_path字段的信息,使得这些信息保持一致。
首先,在Mapper层中定义更新方法:
```java
@Mapper
public interface YourMapper {
void updateProductInfo(@Param("productName") String productName,
@Param("feeUnit") String feeUnit,
@Param("speedUnit") String speedUnit,
@Param("orderAttention") String orderAttention,
@Param("coverImgPath") String coverImgPath);
}
```
接下来,在xml文件中编写SQL语句,用于更新相同产品名称下的信息:
```xml
<!-- YourMapper.xml -->
<mapper namespace="com.example.YourMapper">
<update id="updateProductInfo">
UPDATE your_table
SET feeUnit = #{feeUnit},
speedUnit = #{speedUnit},
order_attention = #{orderAttention},
cover_img_path = #{coverImgPath}
WHERE product_id = (
SELECT product_id
FROM your_table
WHERE product_name = #{productName}
)
</update>
</mapper>
```
然后,在ServiceImpl层中调用Mapper的更新方法:
```java
@Service
public class YourServiceImpl implements YourService {
@Autowired
private YourMapper yourMapper;
@Override
public void updateProductInfo(String productName, String feeUnit, String speedUnit, String orderAttention, String coverImgPath) {
yourMapper.updateProductInfo(productName, feeUnit, speedUnit, orderAttention, coverImgPath);
}
}
```
接下来,在Controller层中调用Service的更新方法:
```java
@RestController
@RequestMapping("/api/products")
public class YourController {
@Autowired
private YourService yourService;
@PutMapping("/{productName}")
public ResponseEntity<String> updateProductInfo(@PathVariable String productName,
@RequestParam String feeUnit,
@RequestParam String speedUnit,
@RequestParam String orderAttention,
@RequestParam String coverImgPath) {
yourService.updateProductInfo(productName, feeUnit, speedUnit, orderAttention, coverImgPath);
return ResponseEntity.ok("Product information updated successfully.");
}
}
```
这样,在调用PUT请求时,通过传递产品名称(productName)和需要更新的字段值(feeUnit、speedUnit、orderAttention、coverImgPath)来更新相同产品名称下的信息,使得这些信息保持一致。
相关推荐
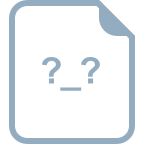














