ndThen(Collectors.
时间: 2024-11-18 21:14:16 浏览: 18
`Collectors.thenCollecting()` 是 Java Stream API 中的一个方法,用于组合多个收集操作(collectors)。它允许你在一系列的收集步骤之后添加额外的操作,比如转换、过滤或者排序。这个方法通常与 `stream().collect()` 结合使用,将多个收集阶段串联起来,提供了一种灵活的方式来构建复杂的数据处理管道。
例如:
```java
List<String> names = ...;
List<Integer> lengths = names.stream()
.map(String::length) // 使用 Collectors.map() 转换每个元素为长度
.thenCollecting(Collectors.toList()); // 将流转换为列表
// 或者,如果有多个收集阶段
Map<String, Integer> countsByLength = names.stream()
.collect(Collectors.groupingBy(String::length))
.thenCollecting(Collectors.toMap(
Map.Entry::getKey, // 第一个函数用于提取键
Map.Entry::getValue, // 第二个函数用于提取值并计数
(oldValue, newValue) -> oldValue + newValue // 函数合并重复的计数值
));
```
相关问题
Collectors.toMap换成Collectors.mapping
Collectors.toMap和Collectors.mapping都是Java 8中的流操作工具类Collectors的方法,用于对流中的元素进行收集和转换。
Collectors.toMap方法用于将流中的元素映射为键值对,并将其收集到一个Map中。它接受两个参数,第一个参数是用于提取键的函数,第二个参数是用于提取值的函数。如果流中存在重复的键,则会抛出一个IllegalStateException异常。
而Collectors.mapping方法则是在收集元素到Map时,对元素进行进一步的转换操作。它接受两个参数,第一个参数是用于提取键的函数,第二个参数是用于对值进行转换的函数。它可以与其他收集器一起使用,例如Collectors.toList或Collectors.toSet,来对值进行进一步的收集和转换。
下面是一个示例代码,演示了如何使用Collectors.toMap和Collectors.mapping方法:
```java
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("apple", "banana", "orange");
// 使用Collectors.toMap将水果列表转换为以长度为键,水果名称为值的Map
Map<Integer, String> lengthToNameMap = fruits.stream()
.collect(Collectors.toMap(String::length, fruit -> fruit));
System.out.println(lengthToNameMap);
// 使用Collectors.mapping将水果列表转换为以长度为键,水果名称列表为值的Map
Map<Integer, List<String>> lengthToNamesMap = fruits.stream()
.collect(Collectors.groupingBy(String::length, Collectors.mapping(fruit -> fruit, Collectors.toList())));
System.out.println(lengthToNamesMap);
}
}
```
输出结果为:
```
{5=apple, 6=orange, 6=banana}
{5=[apple], 6=[orange, banana]}
```
collect方法配合工具类Collectors.toList或者Collectors.toSet或者Collectors.toMap方法,可完成Stream流的数据获取
collect方法是Java Stream API中的重要函数,它允许你在单一流程操作完成后收集数据并转换成特定的形式。当你需要将流中的元素聚集到列表(List)、集合(Set)或映射(Map)中,可以使用 Collectors.toList()、Collectors.toSet() 或 Collectors.toMap() 等工具类。
- Collectors.toList(): 这个工具类用于将流中的所有元素收集到一个List中,元素的顺序通常是按照它们在流中出现的顺序保留的,如果需要有序列表,可以传递一个Comparator作为参数。
- Collectors.toSet(): 该工具会创建一个不可变的Set,自动去除重复元素,适合于不需要顺序并且不允许重复值的情况。
- Collectors.toMap(): 这个工具用于创建一个Map,键和值通常由流中的元素提供。默认情况下,如果键已经存在,旧值会被替换;也可以选择抛出异常(如IllegalStateException)或使用合并策略(如HashMap的putIfAbsent)处理冲突。
使用collect方法的基本语法如下:
```java
List<String> list = streamOfElements.collect(Collectors.toList());
Set<String> set = streamOfElements.collect(Collectors.toSet());
Map<KeyType, ValueType> map = streamOfElements.collect(Collectors.toMap(Function.identity(), Function.identity()));
```
阅读全文
相关推荐
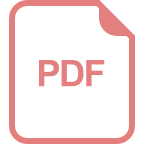
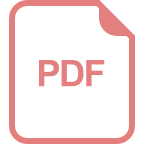
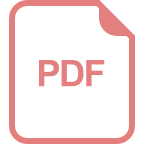













