C语言实现银行业务模拟问题,单队列,多窗口,键盘输入营业时间
时间: 2024-04-12 08:32:33 浏览: 25
您好!对于银行业务模拟问题,可以使用C语言来实现单队列多窗口的模拟。以下是一个简单的示例代码,可以实现基本的银行业务模拟。
```c
#include <stdio.h>
#define MAX_SIZE 100
// 定义队列结构体
typedef struct {
int data[MAX_SIZE];
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue* queue) {
queue->front = 0;
queue->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue* queue) {
return queue->front == queue->rear;
}
// 判断队列是否已满
int isFull(Queue* queue) {
return (queue->rear + 1) % MAX_SIZE == queue->front;
}
// 入队
void enqueue(Queue* queue, int value) {
if (isFull(queue)) {
printf("Queue is full, cannot enqueue.\n");
return;
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % MAX_SIZE;
}
// 出队
int dequeue(Queue* queue) {
if (isEmpty(queue)) {
printf("Queue is empty, cannot dequeue.\n");
return -1; // 表示出错
}
int value = queue->data[queue->front];
queue->front = (queue->front + 1) % MAX_SIZE;
return value;
}
int main() {
int windows;
int duration;
printf("请输入银行窗口数量:");
scanf("%d", &windows);
printf("请输入营业时间(分钟):");
scanf("%d", &duration);
// 初始化多个队列,对应不同的窗口
Queue queues[windows];
for (int i = 0; i < windows; ++i) {
initQueue(&queues[i]);
}
int time = 0;
while (time < duration) {
for (int i = 0; i < windows; ++i) {
if (!isEmpty(&queues[i])) {
printf("窗口%d正在处理顾客:%d\n", i+1, dequeue(&queues[i]));
} else {
printf("窗口%d暂无顾客\n", i+1);
}
}
// 模拟每分钟有一定几率有顾客到来
if (rand() % 4 == 0) {
int window = rand() % windows;
enqueue(&queues[window], time);
printf("顾客到达,排队窗口:%d\n", window+1);
}
time++;
}
return 0;
}
```
这段代码实现了一个简单的银行业务模拟,通过键盘输入窗口数量和营业时间,然后模拟每分钟的业务处理情况。在每分钟内,程序会随机生成顾客到达的情况,并将顾客分配到对应的队列中。然后程序会遍历每个窗口,将正在处理的顾客出队并输出,如果某个窗口没有顾客,则输出"暂无顾客"的提示。请根据实际需求修改代码。
相关推荐




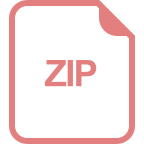
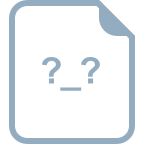
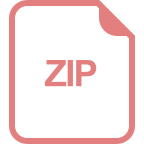
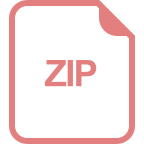