已知某公司员工工龄与平均工资之间有一定的相关关系(见下表),要求使用线性 回归算法分析平均工资与工龄的关系,①使用最小二乘法求解方程并输出W和b值,② 对原始数据与方程的关系图示输出,③并预测工龄为15的平均工资,将预测结果保留 小数点后两位显示输出。 代码
时间: 2024-10-12 20:05:46 浏览: 42
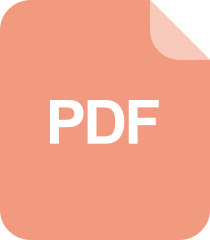
快速数据挖掘数据分析实战RapidMiner工具应用第10章 线性回归与逻辑回归V1.1.pdf

首先,我们需要有实际的数据才能进行线性回归分析。不过,我可以帮你解释一下如何使用Python的`sklearn`库进行这个过程。
1. **导入所需库**:
```python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
```
2. **假设我们有一个DataFrame `df` 包含工龄(years_of_experience)和平均工资(average_salary)列**:
```python
# 假设数据格式如下
data = {
'years_of_experience': [1, 2, 3, ..., 10], # 实际数值填充
'average_salary': [5000, 6000, 7000, ..., 12000] # 实际数值填充
}
df = pd.DataFrame(data)
```
3. **数据预处理**:
- 分割数据集为特征(X)和目标变量(y)
- 划分训练集和测试集
```python
X = df['years_of_experience'].values.reshape(-1, 1) # 形状调整为 (n_samples, n_features)
y = df['average_salary'].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
4. **拟合模型(最小二乘法求解)**:
```python
model = LinearRegression()
model.fit(X_train, y_train)
w = model.coef_[0] # 斜率
b = model.intercept_ # 截距
print(f"W(斜率): {w:.2f}, b(截距): {b:.2f}")
```
5. **绘制散点图与直线**:
```python
plt.scatter(X, y, color='blue')
plt.plot(X, model.predict(X), color='red', label="Linear Regression")
plt.xlabel('工龄')
plt.ylabel('平均工资')
plt.legend()
plt.show()
```
6. **预测工龄为15年的平均工资**:
```python
predicted_salary_15 = model.predict([[15]])[0]
print(f"预测的15年工龄的平均工资: {predicted_salary_15:.2f}")
```
由于没有实际数据,以上步骤都是基于假设的数据结构。你需要替换上述代码中的`...`部分为你实际的数据,并运行它们。
阅读全文
相关推荐
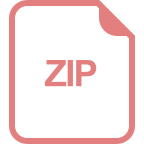
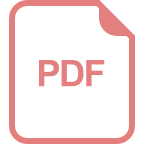



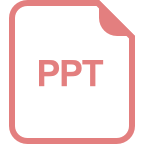
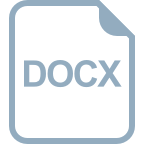
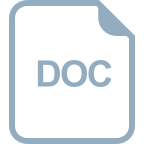
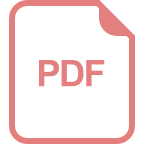
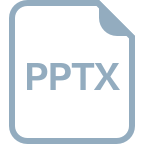
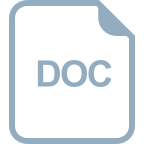
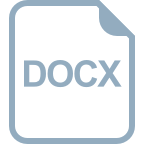
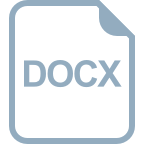
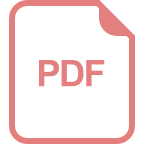
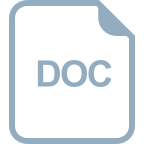
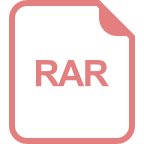