vue未来七天天气弹框
时间: 2024-07-17 16:01:28 浏览: 129
Vue.js是一个流行的前端框架,用于构建用户界面。如果你想在Vue应用中创建一个显示未来七天天气情况的弹框,可以按照以下步骤进行:
1. **安装依赖**:
首先,你需要安装Vue CLI工具来创建项目,然后引入axios库用于获取天气数据,以及可能需要的样式库如Vuetify或Element UI处理弹窗样式。
```sh
npm install -g @vue/cli
vue create your-project-name
cd your-project-name
npm install axios vuetify (或其他你喜欢的UI库)
```
2. **组件设计**:
创建一个名为`WeatherPopup.vue`的新组件,它会包含一个表格或者列表展示日期和对应天气信息。你可以使用v-model绑定状态管理数据,如天气预报数组。
```html
<template>
<v-dialog v-model="showDialog" max-width="500px">
<v-card>
<v-card-title>未来七天天气</v-card-title>
<v-card-content>
<table>
<!-- 表格行数据来自数据绑定 -->
<tr v-for="(weather, index) in forecast" :key="index">
<td>{{ weather.date }}</td>
<td>{{ weather.description }}</td>
</tr>
</table>
</v-card-content>
<v-card-action>
<v-btn text @click="closeDialog">关闭</v-btn>
</v-card-action>
</v-card>
</v-dialog>
</template>
<script>
export default {
data() {
return {
showDialog: false,
forecast: [], // 这里需要从API获取并填充
};
},
methods: {
closeDialog() {
this.showDialog = false;
},
async fetchWeatherData() {
const response = await axios.get('your-api-url');
this.forecast = response.data.slice(0, 7); // 取前7条数据
},
},
mounted() {
this.fetchWeatherData();
},
};
</script>
```
3. **关联到父组件**:
在父组件(如App.vue)中使用v-if或v-show控制这个弹窗的显示,并提供打开和关闭的触发事件。
```html
<template>
<div id="app">
<button @click="openWeatherPopup">查看未来七天天气</button>
<WeatherPopup v-if="showPopup" @close="closePopup"/>
</div>
</template>
<script>
import WeatherPopup from './components/WeatherPopup.vue';
export default {
components: { WeatherPopup },
data() {
return {
showPopup: false,
};
},
methods: {
openWeatherPopup() {
this.showPopup = true;
},
closePopup() {
this.showPopup = false;
},
},
};
</script>
```
相关推荐
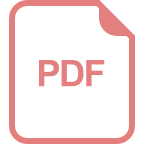
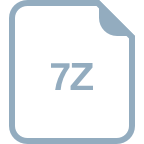














