四维混沌系统加解密Python,实现
时间: 2024-04-06 09:26:53 浏览: 12
四维混沌系统是一种混沌动力学系统,它具有四个状态变量和一组非线性方程。通过对该系统进行加密和解密操作,可以实现信息的保密传输。
在Python中实现四维混沌系统的加解密,可以按照以下步骤进行:
1. 导入所需的库:
```python
import numpy as np
```
2. 定义四维混沌系统的方程:
```python
def four_dimensional_chaos(x, y, z, w, a=10, b=28, c=8/3):
dx = a * (y - x)
dy = x * (b - z) - y
dz = x * y - c * z
dw = -x * w
return dx, dy, dz, dw
```
3. 实现加密函数:
```python
def encrypt(message, key, iterations):
x, y, z, w = key
encrypted_message = ""
for char in message:
for _ in range(iterations):
dx, dy, dz, dw = four_dimensional_chaos(x, y, z, w)
x += dx
y += dy
z += dz
w += dw
encrypted_char = chr(ord(char) ^ int(x))
encrypted_message += encrypted_char
return encrypted_message
```
4. 实现解密函数:
```python
def decrypt(encrypted_message, key, iterations):
x, y, z, w = key
decrypted_message = ""
for char in encrypted_message:
for _ in range(iterations):
dx, dy, dz, dw = four_dimensional_chaos(x, y, z, w)
x += dx
y += dy
z += dz
w += dw
decrypted_char = chr(ord(char) ^ int(x))
decrypted_message += decrypted_char
return decrypted_message
```
使用时,可以调用`encrypt`函数对明文进行加密,调用`decrypt`函数对密文进行解密。其中,`key`参数是四维混沌系统的初始状态,`iterations`参数是迭代次数。
相关推荐
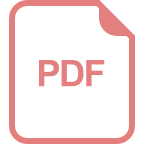
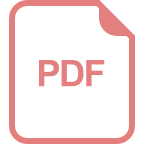
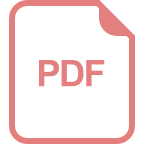














