arduino把char"1234"转化为单个
时间: 2024-09-13 16:17:48 浏览: 32
在Arduino环境中,通常处理的是数字字符而不是文本字符串,比如你想将ASCII码对应的字符数组(如`char array[] = "1234";`)转换为单个整数值,可以逐个字符读取并将它们转换为数字。这可以通过`analogRead()`或其他数字输入方式结合`ASCII`值来实现。
例如,如果你想获取每个字符的ASCII值,可以这样操作:
```c++
const char* input = "1234";
for(int i=0; i<4; i++) {
int digitValue = input[i] - '0'; // 对于数字字符,直接减去 ASCII 码值 '0'
Serial.print(digitValue);
}
```
上述代码会打印出每个字符对应的数字值,注意对于非数字字符,此方法可能会返回错误的结果。
如果你的目标是得到一个整数,你可以先假设所有字符都是数字,然后将其拼接成一个十进制整数:
```c++
int num = 0;
for(int i=0; i<4; i++) {
num = num * 10 + (input[i] - '0'); // 将每一位乘以10,并加当前位的ASCII值
}
Serial.println(num); // 输出整数值,例如:1234
```
相关问题
arduino蓝牙控制单个舵机代码
以下是使用Arduino和蓝牙模块控制单个舵机的示例代码:
```
#include <SoftwareSerial.h>
#include <Servo.h>
SoftwareSerial bluetooth(2, 3); // 配置蓝牙模块的 RX 和 TX 引脚
Servo servo; // 创建舵机对象
void setup() {
bluetooth.begin(9600); // 初始化蓝牙串口通信
servo.attach(9); // 将舵机连接到 9 号引脚
servo.write(90); // 将舵机设置为中间位置
}
void loop() {
if (bluetooth.available()) {
char input = bluetooth.read(); // 读取蓝牙输入
int angle = input - '0'; // 将字符转换为整数
servo.write(angle); // 将舵机旋转到指定角度
}
}
```
在这个示例中,我们使用了SoftwareSerial库来配置蓝牙模块的RX和TX引脚,并且使用了Servo库来控制舵机。我们将舵机连接到Arduino的9号引脚,并将舵机设置为中间位置。在主循环中,我们检查蓝牙是否有数据可用,并将接收到的字符转换为整数。最后,我们将舵机旋转到指定角度。
arduino esp8266传输图片
传输图片需要使用较大的数据量和高速的传输速度,ESP8266模块可以通过WiFi连接到互联网,因此可以使用HTTP协议来传输图片。以下是基本的步骤:
1. 使用Arduino IDE编写程序,包括初始化WiFi连接和HTTP客户端。
2. 打开一个网络连接到图片服务器,通过HTTP GET请求获取图片数据。
3. 将图片数据转换为Base64编码格式,以便在HTTP响应中传输。
4. 将Base64编码的图片数据发送到另一个ESP8266模块或其他设备。
以下是基本的代码示例:
```
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266HTTPClient.h>
#include <Base64.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* serverName = "your_image_server";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Initialize HTTP client
HTTPClient http;
http.begin(serverName);
int httpCode = http.GET();
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
// Convert image data to Base64 encoding
String base64Image = base64::encode(payload);
Serial.println(base64Image);
// Send Base64-encoded image data to another ESP8266 module
// (or another device)
// ...
} else {
Serial.printf("HTTP error code: %d\n", httpCode);
}
http.end();
}
void loop() {
// Do nothing
}
```
请注意,上面的代码示例仅适用于从服务器获取单个图像文件。如果您需要传输多个图像或其他类型的数据,请根据需要修改代码。
阅读全文
相关推荐
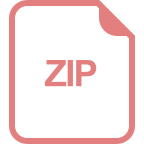
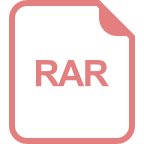
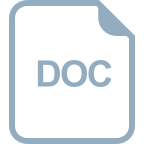
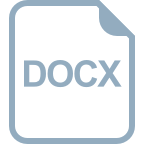
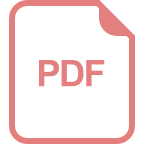
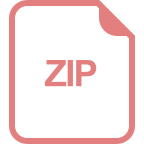
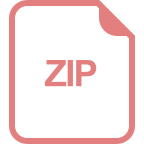
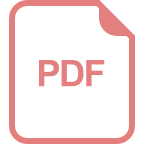
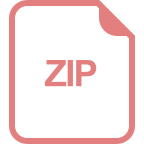
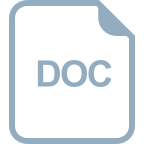
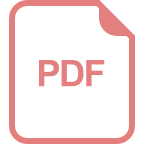
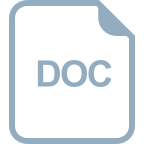
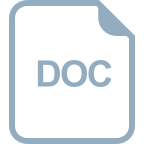



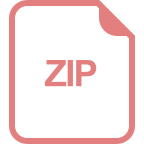