python实现代码,有三个文件夹,两个文件夹中分别存放了一些缺陷图像和图像对应的voc格式的xml标签文件,另外一个文件夹存放了一些正常图像,代码实现提取缺陷图像中的某种名字的标注框,并随机选取一张正常图像粘贴在随机位置中,并形成新的xml标签文件。
时间: 2024-12-08 18:19:11 浏览: 8
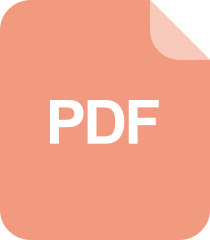
python实现两个文件夹的同步
为了实现这个功能,你可以使用Python的`os`, `shutil`, 和`xml.etree.ElementTree`库来操作文件和XML结构。下面是一个简化的示例代码:
```python
import os
import xml.etree.ElementTree as ET
import random
from PIL import Image
# 定义函数,用于获取某个特定类别(例如"缺陷")的图片和对应的xml文件
def get_defect_images_and_xml(folder_path, category_name):
defect_files = []
for filename in os.listdir(folder_path):
if filename.endswith('.jpg') or filename.endswith('.png'): # 假设是JPG或PNG格式的图像
xml_file = filename.replace('image', 'labels').replace('.jpg', '.xml')
if os.path.isfile(os.path.join(folder_path, xml_file)):
if category_name in xml_file: # 检查xml文件名包含指定类别
defect_files.append((os.path.join(folder_path, filename), os.path.join(folder_path, xml_file)))
return defect_files
# 获取缺陷图片和xml文件
defect_files = get_defect_images_and_xml('缺陷图片文件夹路径', '缺陷')
# 随机选择一张正常图片
normal_folder = '正常图片文件夹路径'
normal_image = random.choice(os.listdir(normal_folder))
# 提取正常图片的宽度和高度
tree = ET.parse(normal_image.replace('.jpg', '.xml'))
root = tree.getroot()
width = int(root.find('size').find('width').text)
height = int(root.find('size').find('height').text)
# 将正常图片随机贴到缺陷图片上并保存
defect_image, defect_xml = defect_files[0] # 只取第一个,这里可以改为随机选择
new_image = Image.open(defect_image).paste(Image.open(normal_image), (random.randint(0, width - normal_image.width), random.randint(0, height - normal_image.height)))
new_image.save('新合成图片.jpg', 'JPEG')
# 创建新的xml标签文件,注意这只是一个基础模板,需要根据实际的xml结构修改
def create_new_xml(old_xml, defect_xml, new_width, new_height):
new_tree = ET.parse(old_xml)
for obj in new_tree.findall('object'):
x_min = int(obj.find('bndbox').find('xmin').text) / new_width
y_min = int(obj.find('bndbox').find('ymin').text) / new_height
x_max = int(obj.find('bndbox').find('xmax').text) / new_width
y_max = int(obj.find('bndbox').find('ymax').text) / new_height
obj.find('bndbox').find('xmin').text = str(x_min)
obj.find('bndbox').find('ymin').text = str(y_min)
obj.find('bndbox').find('xmax').text = str(x_max)
obj.find('bndbox').find('ymax').text = str(y_max)
new_xml_path = '新合成图片.xml'
with open(new_xml_path, 'wb') as f:
f.write(ET.tostring(new_tree, encoding='utf8', method='xml'))
create_new_xml(defect_xml, '合成后的xml路径', width, height)
阅读全文
相关推荐
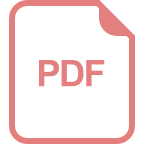
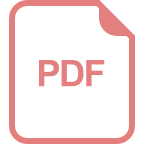
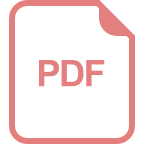
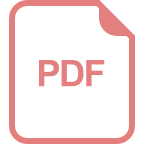
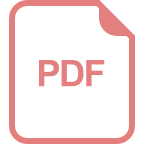
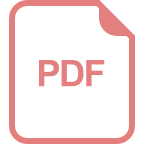
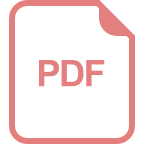
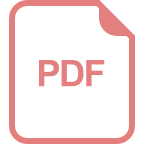
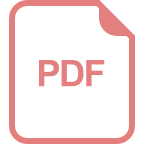
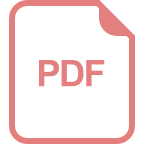
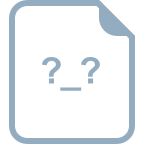
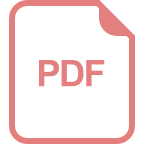
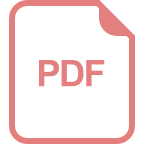