用c语言编写代码,实现用动态和静态2种内存分配方式分别编程实现循环顺序队列的创建、入队、出队、判断队空和队满操作?
时间: 2024-10-08 08:02:07 浏览: 66
在C语言中,我们可以分别使用动态内存分配(malloc/calloc)和静态内存分配(数组)来实现循环顺序队列。以下是两者的简单示例:
**1. 动态内存分配 (链表实现)**
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} QueueNode;
typedef struct {
QueueNode* front; // 队头指针
QueueNode* rear; // 队尾指针
int size; // 队列大小
} Queue;
// 动态内存分配函数
Queue* create_queue(int capacity) {
Queue* q = (Queue*) malloc(sizeof(Queue));
if (!q) {
printf("Memory allocation failed.\n");
return NULL;
}
q->front = q->rear = NULL;
q->size = 0;
return q;
}
// 入队操作
void enqueue(Queue* q, int value) {
if ((q->size + 1) % q->capacity == 0) { // 判断队满
printf("Queue is full.\n");
return;
}
QueueNode* newNode = (QueueNode*) malloc(sizeof(QueueNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = q->rear;
q->rear = newNode;
if (q->front == NULL)
q->front = q->rear;
q->size++;
}
// 出队操作
int dequeue(Queue* q) {
if (q->front == NULL) { // 判断队空
printf("Queue is empty.\n");
return -1;
}
QueueNode* temp = q->front;
int value = temp->data;
q->front = q->front->next;
free(temp);
if (q->front == NULL)
q->rear = NULL;
q->size--;
return value;
}
// 判断队空和队满
int is_empty(Queue* q) {
return q->front == NULL && q->size == 0;
}
int is_full(Queue* q) {
return (q->size + 1) % q->capacity == 0;
}
// 示例用法
int main() {
Queue* dynamic_q = create_queue(5);
// ... 入队、出队操作 ...
return 0;
}
```
**2. 静态内存分配 (数组实现)**
```c
#define QUEUE_SIZE 5
typedef struct {
int queue[QUEUE_SIZE]; // 使用数组存储数据
int front; // 队头索引
int rear; // 队尾索引
} StaticQueue;
StaticQueue* create_queue_static() {
StaticQueue* q = (StaticQueue*) malloc(sizeof(StaticQueue));
q->front = q->rear = -1;
return q;
}
// 入队操作 (静态队列已满无法再入队)
// 出队操作 (静态队列为空无法再出队)
// 判断队空和队满
```
静态队列的入队和出队操作需要额外检查是否溢出边界,这里省略了具体的实现细节。
阅读全文
相关推荐







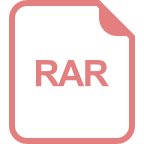










