编写一个程序,运用结构体在自定义函数中完成下列功能并在主函数中进行测试(下列要求中所说的栈均指顺序栈) : (1)初始化栈S。 (2)销毁栈S。 (3)判断栈S是否为空。 (4)将元素e入栈。 (5)将栈顶元素出栈并保存到元素e中。
时间: 2024-10-13 21:15:47 浏览: 33
在C语言中,我们可以创建一个结构体以及相应的栈结构来实现上述功能。首先,我们需要定义一个结构体,例如存储整数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个结构体
typedef struct Node {
int data;
struct Node* next;
} StackElement;
// 初始化栈
StackElement* initStack() {
StackElement* stack = (StackElement*)malloc(sizeof(StackElement));
if (stack == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
stack->next = NULL;
return stack;
}
// 销毁栈
void destroyStack(StackElement* stack) {
StackElement* temp = stack;
while (temp != NULL) {
StackElement* next = temp->next;
free(temp);
temp = next;
}
stack = NULL;
}
// 判断栈是否为空
int isEmpty(StackElement* stack) {
return stack->next == NULL;
}
// 入栈
void push(StackElement** stack, int e) {
StackElement* newNode = (StackElement*)malloc(sizeof(StackElement));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = e;
newNode->next = (*stack)->next;
(*stack)->next = newNode;
}
// 出栈并将栈顶元素保存到e中
int pop(StackElement** stack, int e) {
if (isEmpty(*stack)) {
printf("Stack is empty.\n");
return -1; // 或者抛异常处理
}
StackElement* top = *stack->next;
e = top->data;
(*stack)->next = top->next;
free(top);
return e;
}
// 主函数测试
int main() {
StackElement* stack = initStack();
// 测试操作
if (!isEmpty(stack)) {
printf("Stack initialized successfully.\n");
int e;
push(&stack, 10);
push(&stack, 20);
e = pop(&stack, e); // 应该得到20
printf("Popped element: %d\n", e);
// 释放堆内存
destroyStack(stack);
}
return 0;
}
```
这个示例展示了如何使用结构体和顺序栈来完成指定的功能,并在主函数中进行了简单的测试。注意,在实际应用中,你需要对错误处理进行适当优化。
阅读全文
相关推荐
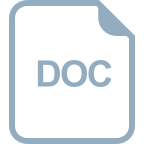
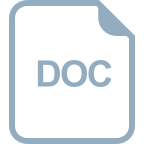
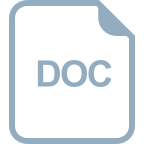







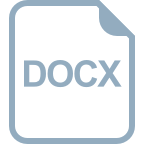






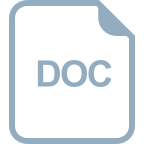
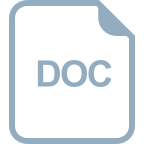