如何使用python操纵外部程序
时间: 2024-11-03 20:12:38 浏览: 18
在Python中,我们可以使用内置的`subprocess`模块来控制其他程序,执行命令行操作。`subprocess`提供了一系列的类和函数来管理子进程,包括运行外部程序、获取输出、检查返回码等。下面是一些常见的使用方法:
1. **使用`Popen`函数**:
```python
import subprocess
process = subprocess.Popen(["cmd", "/c", "your_command"], stdout=subprocess.PIPE, stderr=subprocess.PIPE) # 命令执行
output, error = process.communicate() # 获取输出和错误信息
```
2. **`check_output`函数** (仅在Python 3.5及以上版本可用),它会阻塞直到命令完成,并返回标准输出:
```python
result = subprocess.check_output(["your_command"], text=True) # 输出结果为字符串
```
3. **使用`run`方法** (推荐方法,因为更简洁且自动处理一些异常):
```python
result = subprocess.run(["your_command"], capture_output=True, text=True)
print(result.stdout) # 输出结果
```
4. **输入和输出重定向**:
```python
with open('input.txt', 'r') as f:
process = subprocess.Popen(['program', '-i'], stdin=f, stdout=subprocess.PIPE)
```
5. **异步执行**:
如果需要异步执行,可以使用`subprocess.Popen`的`start_new_session`选项开启一个新的子进程组(session):
```python
process = subprocess.Popen(["your_command"], start_new_session=True)
```
阅读全文
相关推荐
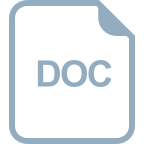
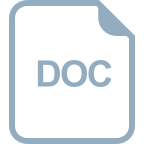
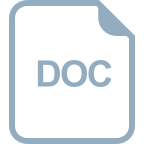
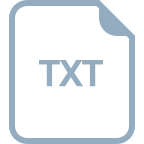
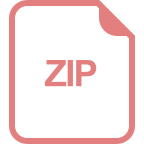
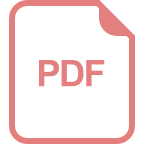
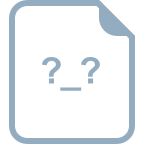
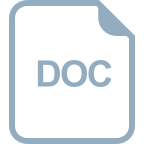
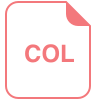
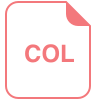
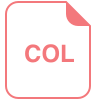
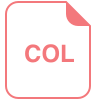
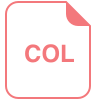
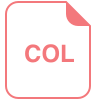
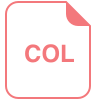
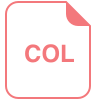
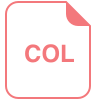
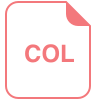