进程死锁(银行家算法)完整C语言代码
时间: 2024-10-17 18:09:47 浏览: 100
进程死锁是指两个或更多的进程各自占有某种资源而又等待对方所占有的资源,导致它们都无法继续执行的一种状态。银行家算法是一种避免死锁的发生,用于动态分配系统资源的并发控制策略。
以下是基于银行家算法的一个简化版的C语言代码示例,它主要用于四个请求者进程共享四种类型的资源:
```c
#include <stdio.h>
#define MAX_PROCESSES 4
#define MAX_RESOURCES 4
typedef struct {
int pid; // Process ID
int demand[MAX_RESOURCES]; // Requested resources
int allocation[MAX_RESOURCES]; // Allocated resources
int ready; // Ready to request more resources
} Process;
int AvailableResources[MAX_RESOURCES];
int NoOfProcess;
// Function to check if system will be in safe state after the given request
int SafeState(int* request, int maxRequest) {
for (int i = 0; i < MAX_RESOURCES; ++i) {
if (request[i] + AvailableResources[i] >= maxRequest[i]) return 0;
}
return 1;
}
// Banker's algorithm function
void BankersAlgorithm() {
while (1) {
// Find a process that is blocked due to resource starvation
int deadlockDetected = 0;
for (int p = 0; p < NoOfProcess && !deadlockDetected; ++p) {
if (!process[p].ready && IsDeadlock(process[p], AvailableResources)) {
deadlockDetected = 1;
printf("Deadlock detected for process %d\n", process[p].pid);
}
}
// If no deadlock found, check if we can make some processes ready
if (!deadlockDetected) {
for (int p = 0; p < NoOfProcess; ++p) {
if (!process[p].ready) {
if (SafeState(&process[p].demand[process[p].allocation], process[p].maxRequest)) {
process[p].ready = 1;
printf("Process %d made ready with current allocation: ", process[p].pid);
PrintAllocation();
}
}
}
}
else {
// Deadlock recovery (e.g., killing one or more processes)
// In this simple example, it's skipped
break;
}
}
}
// ... (Add helper functions like IsDeadlock(), PrintAllocation(), etc.)
int main() {
// Initialize processes and available resources
// ... (Your initialization code here)
// Run the bank家算法
BankersAlgorithm();
return 0;
}
```
请注意,这只是一个简化的示例,并未包含死锁恢复的部分以及完整的用户输入处理。在实际应用中,你还需要设计更详细的函数来管理请求、资源分配和死锁检测。
阅读全文
相关推荐



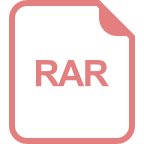












