输入num1+Operator+num2各式的表达式+已知num1和num2都为整数,如果per+at+or为+.-.*./和%一种+请编写程序+设计这个计算器
时间: 2024-01-02 22:02:52 浏览: 29
为了设计这个计算器,你需要遵循以下步骤:
1. 首先,你需要定义一个函数来解析输入的表达式并计算结果。这个函数应该接受一个字符串参数,表示输入的表达式,并返回一个整数结果。
2. 在解析表达式之前,你需要将表达式中的空格和其他无用字符去掉,只保留数字和运算符。
3. 接下来,你需要将表达式分解成数字和运算符的序列。你可以使用一个栈来实现这个过程。遍历表达式中的每个字符,如果是数字则将其压入栈中,如果是运算符则弹出栈顶的两个数字进行计算,并将计算结果压入栈中。
4. 最后,当遍历完整个表达式后,栈中只剩下一个数字,这个数字就是表达式的计算结果。
5. 你还需要考虑一些特殊情况,例如除数为0的情况和输入表达式不合法的情况。
下面是一个示例代码,可以实现上述计算器的功能:
```
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int calculate(string expression) {
stack<int> nums;
stack<char> ops;
for (int i = 0; i < expression.length(); i++) {
if (expression[i] == ' ') {
continue;
} else if (isdigit(expression[i])) {
int num = 0;
while (i < expression.length() && isdigit(expression[i])) {
num = num * 10 + (expression[i] - '0');
i++;
}
i--;
nums.push(num);
} else if (expression[i] == '+' || expression[i] == '-' || expression[i] == '*' || expression[i] == '/' || expression[i] == '%') {
while (!ops.empty() && (ops.top() == '*' || ops.top() == '/' || ops.top() == '%')) {
int num2 = nums.top();
nums.pop();
int num1 = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '*') {
nums.push(num1 * num2);
} else if (op == '/') {
if (num2 == 0) {
throw "Divide by zero error";
}
nums.push(num1 / num2);
} else if (op == '%') {
if (num2 == 0) {
throw "Divide by zero error";
}
nums.push(num1 % num2);
}
}
ops.push(expression[i]);
} else {
throw "Invalid input";
}
}
while (!ops.empty()) {
int num2 = nums.top();
nums.pop();
int num1 = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '+') {
nums.push(num1 + num2);
} else if (op == '-') {
nums.push(num1 - num2);
} else if (op == '*') {
nums.push(num1 * num2);
} else if (op == '/') {
if (num2 == 0) {
throw "Divide by zero error";
}
nums.push(num1 / num2);
} else if (op == '%') {
if (num2 == 0) {
throw "Divide by zero error";
}
nums.push(num1 % num2);
}
}
if (nums.size() != 1) {
throw "Invalid input";
}
return nums.top();
}
int main() {
string expression;
cout << "Please enter an expression: ";
getline(cin, expression);
try {
int result = calculate(expression);
cout << "Result: " << result << endl;
} catch (const char* msg) {
cerr << "Error: " << msg << endl;
}
return 0;
}
```
相关推荐
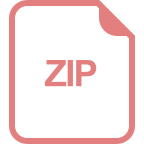
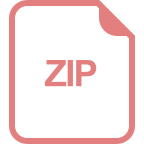
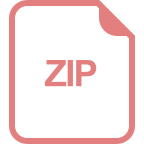














