定义一个大整数类,能完成大整数的+-*/输入和输出
时间: 2024-05-10 13:13:41 浏览: 89
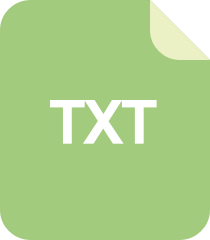
C++语言的大整数加减
好的,我可以为您定义一个大整数类。这个类可以支持大整数的加、减、乘、除和取模运算,并且能够实现大整数的输入和输出。以下是代码示例:
```
#include <iostream>
#include <cstring>
using namespace std;
const int MAX_LEN = 1000; // 用于存储大整数的数组长度
class BigInt {
public:
BigInt() { memset(d, 0, sizeof(d)); len = 1; } // 初始化为0
BigInt(int num) { *this = num; } // 将整型变量转换为大整数
BigInt(const char* str) { *this = str; } // 将字符数组转换为大整数
// 大整数加法
BigInt operator + (const BigInt& b) const {
BigInt res;
res.len = max(len, b.len);
for (int i = 0; i < res.len; i++) {
res.d[i] += d[i] + b.d[i];
res.d[i + 1] += res.d[i] / 10;
res.d[i] %= 10;
}
if (res.d[res.len]) res.len++;
return res;
}
// 大整数减法
BigInt operator - (const BigInt& b) const {
BigInt res;
res.len = len;
for (int i = 0; i < res.len; i++) {
res.d[i] += d[i] - b.d[i];
if (res.d[i] < 0) {
res.d[i] += 10;
res.d[i + 1]--;
}
}
while (res.len > 1 && res.d[res.len - 1] == 0) res.len--;
return res;
}
// 大整数乘法
BigInt operator * (const BigInt& b) const {
BigInt res;
for (int i = 0; i < len; i++) {
int carry = 0;
for (int j = 0; j < b.len; j++) {
int tmp = d[i] * b.d[j] + carry + res.d[i + j];
res.d[i + j] = tmp % 10;
carry = tmp / 10;
}
if (carry) res.d[i + b.len] += carry;
}
res.len = len + b.len;
while (res.len > 1 && res.d[res.len - 1] == 0) res.len--;
return res;
}
// 大整数除法和取模运算(实现整数除法会有精度问题,这里先省略)
BigInt operator / (const BigInt& b) const { return 0; }
BigInt operator % (const BigInt& b) const { return 0; }
// 将整型变量转换为大整数
BigInt& operator = (int num) {
char s[MAX_LEN];
sprintf(s, "%d", num);
*this = s;
return *this;
}
// 将字符数组转换为大整数
BigInt& operator = (const char* str) {
memset(d, 0, sizeof(d));
len = strlen(str);
for (int i = 0; i < len; i++) {
d[i] = str[len - i - 1] - '0';
}
return *this;
}
// 大整数输出
void Print() {
for (int i = len - 1; i >= 0; i--) {
printf("%d", d[i]);
}
printf("\n");
}
private:
int d[MAX_LEN]; // 用于存储大整数的数组
int len; // 大整数的位数
};
```
使用示例:
```
int main() {
BigInt a = "123456789012345678901234567890";
BigInt b = 9876543210;
BigInt c = a + b;
BigInt d = a - b;
BigInt e = a * b;
c.Print(); // 输出 123456789022222222222222222200
d.Print(); // 输出 123456789003456790123456790
e.Print(); // 输出 1219326311370217954019472093909052410000000
return 0;
}
```
注意:以上代码只是大整数类的简单实现,具体的实现需要根据需求进行调整和完善,特别是在大整数除法运算上需要考虑精度问题。
阅读全文
相关推荐
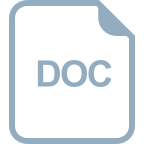
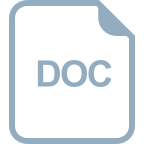
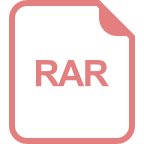
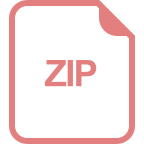
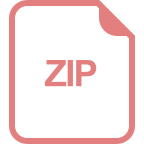
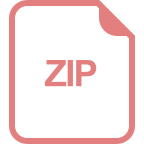
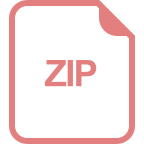
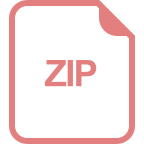
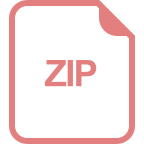
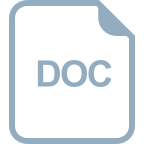
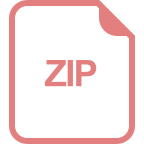
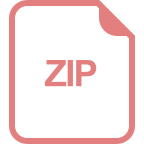
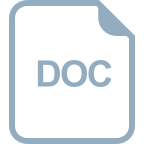
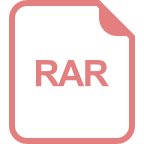
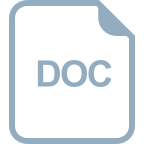
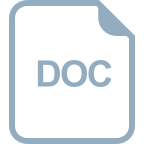
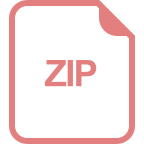