大一c语言学生管理系统
时间: 2023-12-22 08:28:41 浏览: 135
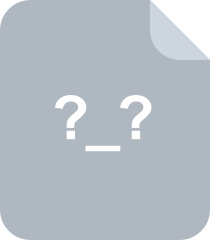
c语言学生管理系统
大一C语言学生管理系统可以包括以下功能:
1. 录入学生信息:包括学号、姓名和各科考试成绩。
2. 计算每门课程的总分和平均分:根据学生的成绩计算每门课程的总分和平均分。
3. 计算每个学生的总分和平均分:根据学生的成绩计算每个学生的总分和平均分。
4. 排名功能:按照每个学生的总分由高到低或由低到高排出名次表。
5. 学号排序功能:按照学号由小到大或由大到小排出名次表。
以下是一个示例代码,实现了上述功能:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STUDENTS 100
#define MAX_NAME_LENGTH 50
#define MAX_SUBJECTS 5
struct Student {
int id;
char name[MAX_NAME_LENGTH];
int scores[MAX_SUBJECTS];
int totalScore;
float averageScore;
};
void inputStudents(struct Student students[], int numStudents) {
for (int i = 0; i < numStudents; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%d", &students[i].id);
printf("请输入第%d个学生的姓名:", i + 1);
scanf("%s", students[i].name);
printf("请输入第%d个学生的各科成绩:\n", i + 1);
for (int j = 0; j < MAX_SUBJECTS; j++) {
printf("请输入第%d门课的成绩:", j + 1);
scanf("%d", &students[i].scores[j]);
}
}
}
void calculateSubjectScores(struct Student students[], int numStudents) {
for (int i = 0; i < MAX_SUBJECTS; i++) {
int totalScore = 0;
for (int j = 0; j < numStudents; j++) {
totalScore += students[j].scores[i];
}
printf("第%d门课的总分:%d,平均分:%.2f\n", i + 1, totalScore, (float)totalScore / numStudents);
}
}
void calculateStudentScores(struct Student students[], int numStudents) {
for (int i = 0; i < numStudents; i++) {
int totalScore = 0;
for (int j = 0; j < MAX_SUBJECTS; j++) {
totalScore += students[i].scores[j];
}
students[i].totalScore = totalScore;
students[i].averageScore = (float)totalScore / MAX_SUBJECTS;
printf("%s的总分:%d,平均分:%.2f\n", students[i].name, totalScore, students[i].averageScore);
}
}
void sortByTotalScore(struct Student students[], int numStudents, int ascending) {
for (int i = 0; i < numStudents - 1; i++) {
for (int j = 0; j < numStudents - i - 1; j++) {
if ((ascending && students[j].totalScore > students[j + 1].totalScore) ||
(!ascending && students[j].totalScore < students[j + 1].totalScore)) {
struct Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
}
void sortByStudentId(struct Student students[], int numStudents, int ascending) {
for (int i = 0; i < numStudents - 1; i++) {
for (int j = 0; j < numStudents - i - 1; j++) {
if ((ascending && students[j].id > students[j + 1].id) ||
(!ascending && students[j].id < students[j + 1].id)) {
struct Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp; }
}
}
}
int main() {
struct Student students[MAX_STUDENTS];
int numStudents;
printf("请输入学生人数:");
scanf("%d", &numStudents);
inputStudents(students, numStudents);
calculateSubjectScores(students, numStudents);
calculateStudentScores(students, numStudents);
sortByTotalScore(students, numStudents, 0); // 按总分由高到低排序
printf("按总分由高到低排名表:\n");
for (int i = 0; i < numStudents; i++) {
printf("第%d名:%s,总分:%d\n", i + 1, students[i].name, students[i].totalScore);
}
sortByTotalScore(students, numStudents, 1); // 按总分由低到高排序
printf("按总分由低到高排名表:\n");
for (int i = 0; i < numStudents; i++) {
printf("第%d名:%s,总分:%d\n", i + 1, students[i].name, students[i].totalScore);
}
sortByStudentId(students, numStudents, 1); // 按学号由小到大排序
printf("按学号由小到大排名表:\n");
for (int i = 0; i < numStudents; i++) {
printf("第%d名:%s,学号:%d\n", i + 1, students[i].name, students[i].id);
}
sortByStudentId(students, numStudents, 0); // 按学号由大到小排序
printf("按学号由大到小排名表:\n");
for (int i = 0; i < numStudents; i++) {
printf("第%d名:%s,学号:%d\n", i + 1, students[i].name, students[i].id);
}
return 0;
}
```
阅读全文
相关推荐









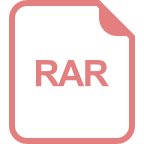
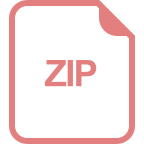
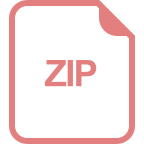
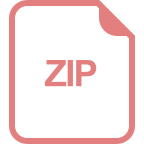
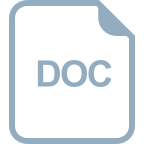
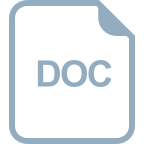