c语言实现对字符串加密 不使用凯撒加密
时间: 2024-10-17 12:08:20 浏览: 22
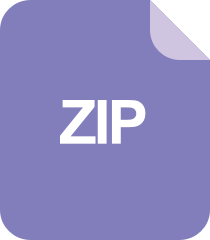
凯撒加密算法的C语言实现.zip
在C语言中,除了传统的凯撒加密外,还有许多其他加密算法可以应用于字符串,如简单的位移(异或)、替换字典或其他更复杂的加密算法(如MD5、SHA-1等,但它们通常不是用于日常文本加密,而是用于数据完整性校验或高级加密)。这里以简单的位移(异或操作)为例,它不属于现代加密标准,但可用于演示基础加密原理。
```c
#include <stdio.h>
#include <string.h>
// 对字符串中的每个字符进行异或操作
void xor_encrypt(char* plaintext, char* key) {
int len = strlen(plaintext);
for (int i = 0; i < len; i++) {
plaintext[i] ^= key[i % strlen(key)]; // 字符异或操作
}
}
void decrypt_with_key(char* encrypted_text, char* key) {
xor_encrypt(encrypted_text, key); // 解密时也做同样的异或操作
printf("解密后的字符串: %s\n", encrypted_text);
}
int main() {
char plaintext[100], key[100];
printf("请输入明文: ");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strcspn(plaintext, "\n")] = '\0';
printf("请输入密钥: ");
fgets(key, sizeof(key), stdin);
key[strcspn(key, "\n")] = '\0'; // 移除换行符
xor_encrypt(plaintext, key); // 加密
printf("加密后的字符串: %s\n", plaintext);
decrypt_with_key(plaintext, key); // 解密(实际上是反加密)
return 0;
}
```
在这个例子中,我们首先读取明文和密钥,然后使用异或操作对每个字符进行加密。解密时只需再做一次相同的异或操作即可恢复原始字符串。
阅读全文
相关推荐
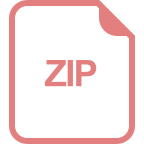
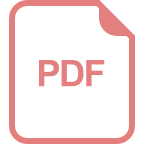















