用C语言加密凯撒密码
时间: 2024-10-09 15:16:26 浏览: 42
凯撒密码是一种简单的替换式加密方法,它通过将明文中的每个字母按照固定位移(密钥)进行替换来实现加密。在C语言中实现凯撒密码,通常涉及到字符数组的操作和循环。以下是一个基本的凯撒密码加密算法示例,这里我们假设密钥是固定的,例如3:
```c
#include <stdio.h>
#include <string.h>
char caesar_encrypt(char* plaintext, int key) {
int len = strlen(plaintext);
char encrypted[len];
for (int i = 0; i < len; i++) {
// 对大写字母、小写字母分别处理
if ('a' <= plaintext[i] && plaintext[i] <= 'z') {
encrypted[i] = ((plaintext[i] - 'a' + key) % 26) + 'a';
} else if ('A' <= plaintext[i] && plaintext[i] <= 'Z') {
encrypted[i] = ((plaintext[i] - 'A' + key) % 26) + 'A';
} else { // 如果不是字母,则保持原样
encrypted[i] = plaintext[i];
}
}
return encrypted;
}
int main() {
char message[] = "Hello, World!";
int key = 3;
char encrypted_message[50];
strcpy(encrypted_message, caesar_encrypt(message, key));
printf("Original Message: %s\n", message);
printf("Encrypted Message: %s\n", encrypted_message);
return 0;
}
```
在这个例子中,`caesar_encrypt`函数接受一个字符指针和一个密钥,然后遍历输入的字符串,对每个字母进行相应的移动并替换。`main`函数则展示了如何使用这个加密函数。
阅读全文
相关推荐
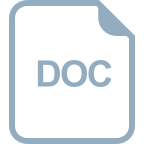
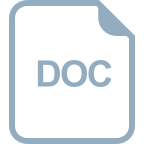
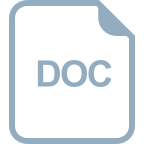

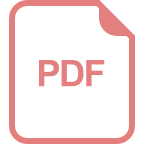
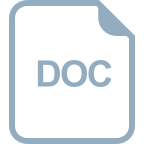
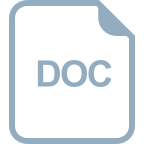
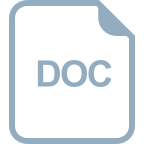
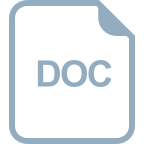
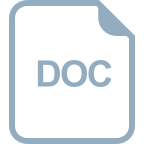






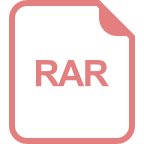
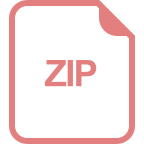