c语言实现凯撒密码加密julus
时间: 2024-11-15 17:21:40 浏览: 5
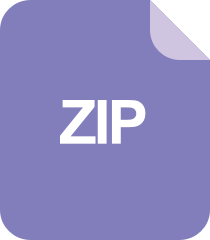
RuoYi-Vue 全新 Pro 版本,优化重构所有功能
凯撒密码是一种简单的替换式加密方法,它通过将明文字符按照字母表顺序向后(或者向前)移动固定数量的位置来进行加密。在C语言中实现凯撒密码,可以创建一个函数,接受输入字符串、偏移量以及是否大写处理作为参数。以下是基本的步骤:
```c
#include <stdio.h>
#include <string.h>
char caesar_encrypt(char ch, int shift, bool is_uppercase) {
if (is_uppercase && ch >= 'A' && ch <= 'Z') {
return ((ch - 'A' + shift) % 26) + 'A';
} else if (!is_uppercase && ch >= 'a' && ch <= 'z') {
return ((ch - 'a' + shift) % 26) + 'a';
}
// 非字母字符保持原样
return ch;
}
void caesar_cipher(const char *plaintext, int shift, char encrypted[], const char alphabet[58]) {
for (int i = 0; plaintext[i] != '\0'; i++) {
encrypted[i] = caesar_encrypt(plaintext[i], shift, isupper(plaintext[i]));
}
encrypted[i] = '\0'; // 添加终止符
}
int main() {
const char plaintext[] = "Hello, World!";
char encrypted[100];
int shift = 3; // 示例偏移量
caesar_cipher(plaintext, shift, encrypted, "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz");
printf("原文: %s\n", plaintext);
printf("密文: %s\n", encrypted);
return 0;
}
```
在这个示例中,`caesar_encrypt` 函数用于单个字符的加密,`caesar_cipher` 函数遍历整个字符串并应用加密。注意这里假设了只有字母会被替换,并且我们创建了一个包含所有大小写字母的小写字母表(`alphabet`)。运行这个程序,你可以看到输入字符串"Hello, World!"经过3位偏移后的加密结果。
阅读全文
相关推荐
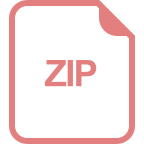
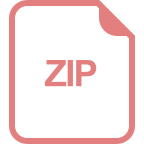
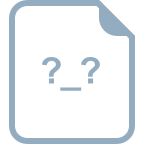
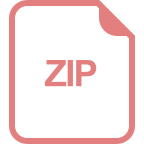
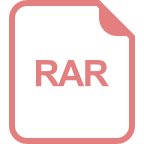
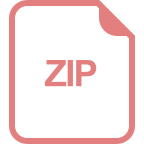
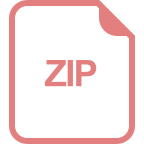
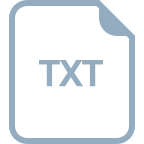
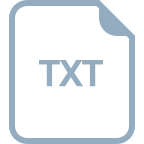