如何避免类似问题,确保Tkinter操作在主线程执行?
时间: 2024-10-18 08:23:38 浏览: 61
为了避免Tkinter操作在非主线程引发的`RuntimeError`,你可以采取以下几种策略:
1. **同步操作**: 使用`tkinter`的`after`或`update`方法在主线程中安排回调。例如,在子线程完成后,可以设置一个定时器或事件来更新主线程的任务列表,然后调用`root.update()`或`root.after(milliseconds, callback)`来触发GUI更新。
```python
import tkinter as tk
from threading import Thread
def update_gui():
# 这里的操作应该放在主线程的回调函数里
root.showinfo("提示", "任务完成")
# 子线程执行
def worker():
# 子线程代码...
# ...
# 执行完毕后调用回调
root.after(0, update_gui)
# 创建主线程
root = tk.Tk()
# 启动子线程
worker_thread = Thread(target=worker)
worker_thread.start()
# 等待子线程结束
worker_thread.join()
```
2. **`join`方法配合`:}`**: 结束线程时确保其完成并返回到主线程。这可以通过在子线程内部使用`join()`,并在退出时清理Tkinter实例来实现。
```python
import tkinter as tk
from threading import Thread
def worker(root):
# 子线程代码...
# ...
root.quit() # 让主线程结束
root = tk.Tk()
thread = Thread(target=worker, args=(root,))
thread.start()
thread.join() # 确保线程结束后再关闭窗口
root.destroy()
```
3. **`concurrent.futures`库**: 如果有大量异步任务,可以使用`ThreadPoolExecutor`结合`Future`来处理,它会自动在适当的时机将结果传递给主线程。
```python
from concurrent.futures import ThreadPoolExecutor, Future
def task_runner(future):
# 子线程任务
future.set_result(result)
executor = ThreadPoolExecutor()
future = executor.submit(task)
future.result() # 当任务完成时,这里会在主线程中获取结果
```
阅读全文
相关推荐
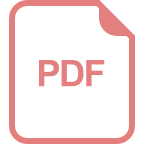
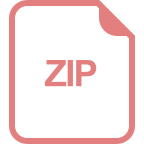
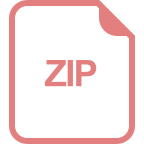
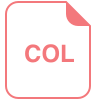
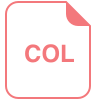
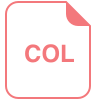
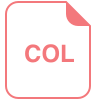
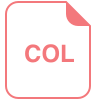
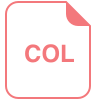
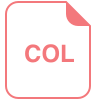
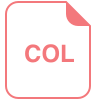
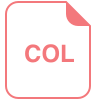
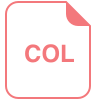
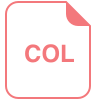
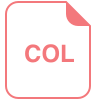
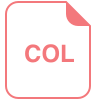
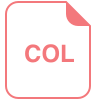
