python的正则匹配并替换
时间: 2023-10-18 17:30:48 浏览: 136
你可以使用 `re` 模块来进行正则匹配和替换。下面是一个示例代码:
```python
import re
text = "Hello, my name is John. I love Python."
# 使用正则表达式查找名字并替换为"Tom"
new_text = re.sub(r'John', 'Tom', text)
print(new_text)
```
输出结果为:
```
Hello, my name is Tom. I love Python.
```
在这个例子中,我们使用了 `re.sub()` 函数来匹配并替换字符串中的模式。第一个参数是要匹配的正则表达式模式,第二个参数是要替换的字符串,第三个参数是要进行匹配和替换的原始文本。
相关问题
python 正则匹配
Python正则表达式是一种强大的工具,可以用于字符串匹配、搜索和替换。以下是Python中使用正则表达式的基本步骤:
1.导入re模块
```
import re
```
2.使用re.compile()函数创建正则表达式对象
```
pattern = re.compile(r'正则表达式')
```
3.使用正则表达式匹配字符串
```
match_result = pattern.match(string)
search_result = pattern.search(string)
findall_result = pattern.findall(string)
```
4.对匹配结果进行操作
```
match_result.group() # 返回匹配的字符串
match_result.span() # 返回匹配的起始和结束位置
```
示例:
```
import re
# 创建正则表达式对象
pattern = re.compile(r'\d+')
# 匹配字符串
match_result = pattern.match('12345')
search_result = pattern.search('hello 12345')
findall_result = pattern.findall('hello 12345 world 67890')
# 对匹配结果进行操作
print(match_result.group()) # 输出: 12345
print(search_result.group()) # 输出: 12345
print(findall_result) # 输出: ['12345', '67890']
```
以上是Python正则表达式的基本用法,更多高级功能请参考官方文档。
python的正则匹配替换
你可以使用 `re` 模块来进行正则匹配和替换。下面是一个示例代码:
```python
import re
text = "Hello, my name is John. I love Python."
# 使用正则表达式查找名字并替换为"Tom"
new_text = re.sub(r'John', 'Tom', text)
print(new_text)
```
输出结果为:
```
Hello, my name is Tom. I love Python.
```
在这个例子中,我们使用了 `re.sub()` 函数来匹配并替换字符串中的模式。第一个参数是要匹配的正则表达式模式,第二个参数是要替换的字符串,第三个参数是要进行匹配和替换的原始文本。
阅读全文
相关推荐
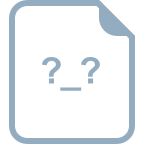
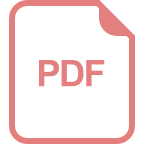
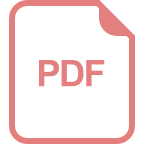
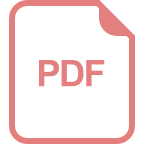
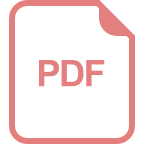
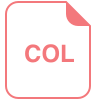









