springboot中ftp连接池
时间: 2023-08-10 10:00:26 浏览: 66
Spring Boot中可以通过使用Apache Commons Net库来实现FTP连接池。下面是实现FTP连接池的步骤和代码示例:
1. 首先,需要在pom.xml文件中添加Apache Commons Net依赖:
```xml
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. 创建一个FTP连接池管理类,用于管理和维护FTP连接池。可以使用Apache Commons Pool库来实现连接池的功能:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.pool2.ObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPool;
public class FTPPoolManager {
private static ObjectPool<FTPClient> pool;
static {
// 创建FTPClientFactory实例,用于创建FTPClient对象
FTPClientFactory factory = new FTPClientFactory();
// 配置连接池
GenericObjectPool.Config config = new GenericObjectPool.Config();
config.setMaxTotal(10); // 设置连接池最大连接数
config.setMaxIdle(5); // 设置连接池最大空闲连接数
config.setMinIdle(1); // 设置连接池最小空闲连接数
// 创建连接池
pool = new GenericObjectPool<>(factory, config);
}
public static FTPClient borrowObject() throws Exception {
return pool.borrowObject();
}
public static void returnObject(FTPClient ftpClient) {
pool.returnObject(ftpClient);
}
}
```
3. 创建一个FTPClient工厂类,用于创建FTPClient对象:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.pool2.BasePooledObjectFactory;
public class FTPClientFactory extends BasePooledObjectFactory<FTPClient> {
@Override
public FTPClient create() throws Exception {
// 创建FTPClient对象
FTPClient ftpClient = new FTPClient();
ftpClient.connect("ftp.example.com", 21); // 连接FTP服务器
ftpClient.login("username", "password"); // 登录FTP服务器
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE); // 设置传输模式为二进制
return ftpClient;
}
@Override
public void destroyObject(FTPClient ftpClient) throws Exception {
ftpClient.logout(); // 退出登录
ftpClient.disconnect(); // 断开连接
}
@Override
public boolean validateObject(FTPClient ftpClient) {
boolean result = false;
try {
result = ftpClient.sendNoOp(); // 发送NOOP命令检测连接是否有效
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
```
4. 在使用FTP连接的地方,可以通过FTP连接池管理类来获取FTPClient对象,并使用完后归还给连接池:
```java
import org.apache.commons.net.ftp.FTPClient;
public class FTPExample {
public static void main(String[] args) {
FTPClient ftpClient = null;
try {
ftpClient = FTPPoolManager.borrowObject(); // 从连接池中获取FTPClient对象
// 在这里执行FTP操作,如上传文件、下载文件等
} catch (Exception e) {
e.printStackTrace();
} finally {
if (ftpClient != null) {
FTPPoolManager.returnObject(ftpClient); // 将FTPClient对象归还给连接池
}
}
}
}
```
通过以上步骤和代码示例,我们可以在Spring Boot中实现FTP连接池,以提高FTP连接的性能和效率。
相关推荐
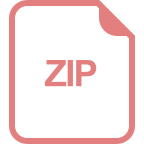














