springboot ftp连接池实现
时间: 2023-11-22 12:48:03 浏览: 140
以下是使用SpringBoot实现FTP连接池的步骤:
1.在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2.创建FTP连接池配置类FtpClientPoolConfig,配置连接池的最大、最小连接数、连接超时时间等参数。
```java
@Configuration
@ConfigurationProperties(prefix = "ftp.pool")
@Data
public class FtpClientPoolConfig {
private int maxTotal;
private int maxIdle;
private int minIdle;
private long maxWaitMillis;
private boolean testOnBorrow;
private boolean testOnReturn;
private boolean testWhileIdle;
private long timeBetweenEvictionRunsMillis;
private int numTestsPerEvictionRun;
private long minEvictableIdleTimeMillis;
}
```
3.创建FTP连接池类FtpClientPool,使用Apache Commons Pool2实现连接池。
```java
@Component
public class FtpClientPool extends GenericObjectPool<FtpClient> {
public FtpClientPool(FtpClientFactory factory, FtpClientPoolConfig config) {
super(factory, new GenericObjectPoolConfig());
this.setMaxTotal(config.getMaxTotal());
this.setMaxIdle(config.getMaxIdle());
this.setMinIdle(config.getMinIdle());
this.setMaxWaitMillis(config.getMaxWaitMillis());
this.setTestOnBorrow(config.isTestOnBorrow());
this.setTestOnReturn(config.isTestOnReturn());
this.setTestWhileIdle(config.isTestWhileIdle());
this.setTimeBetweenEvictionRunsMillis(config.getTimeBetweenEvictionRunsMillis());
this.setNumTestsPerEvictionRun(config.getNumTestsPerEvictionRun());
this.setMinEvictableIdleTimeMillis(config.getMinEvictableIdleTimeMillis());
}
}
```
4.创建FTP连接池工厂类FtpClientFactory,用于创建FTP连接对象。
```java
@Component
public class FtpClientFactory extends BasePooledObjectFactory<FtpClient> {
private FtpClientProperties properties;
public FtpClientFactory(FtpClientProperties properties) {
this.properties = properties;
}
@Override
public FtpClient create() throws Exception {
FtpClient ftpClient = new FtpClient();
ftpClient.connect(properties.getHost(), properties.getPort());
ftpClient.login(properties.getUsername(), properties.getPassword());
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
ftpClient.setBufferSize(properties.getBufferSize());
ftpClient.setControlEncoding(properties.getEncoding());
ftpClient.enterLocalPassiveMode();
return ftpClient;
}
@Override
public PooledObject<FtpClient> wrap(FtpClient ftpClient) {
return new DefaultPooledObject<>(ftpClient);
}
@Override
public void destroyObject(PooledObject<FtpClient> p) throws Exception {
FtpClient ftpClient = p.getObject();
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
@Override
public boolean validateObject(PooledObject<FtpClient> p) {
FtpClient ftpClient = p.getObject();
try {
return ftpClient.sendNoOp();
} catch (IOException e) {
return false;
}
}
}
```
5.创建FTP连接池属性类FtpClientProperties,用于配置FTP连接的相关参数。
```java
@ConfigurationProperties(prefix = "ftp")
@Data
public class FtpClientProperties {
private String host;
private int port;
private String username;
private String password;
private int bufferSize;
private String encoding;
}
```
6.在application.yml文件中配置FTP连接池的相关参数。
```yaml
ftp:
host: ftp.example.com
port: 21
username: username
password: password
bufferSize: 1024
encoding: UTF-8
ftp:
pool:
maxTotal: 10
maxIdle: 5
minIdle: 1
maxWaitMillis: 3000
testOnBorrow: true
testOnReturn: false
testWhileIdle: true
timeBetweenEvictionRunsMillis: 60000
numTestsPerEvictionRun: -1
minEvictableIdleTimeMillis: 1800000
```
7.在需要使用FTP连接的地方,注入FtpClientPool对象,从连接池中获取FTP连接对象。
```java
@Service
public class FtpService {
@Autowired
private FtpClientPool ftpClientPool;
public void uploadFile(String remotePath, String fileName, InputStream inputStream) throws Exception {
FtpClient ftpClient = ftpClientPool.borrowObject();
try {
ftpClient.changeWorkingDirectory(remotePath);
ftpClient.storeFile(fileName, inputStream);
} finally {
ftpClientPool.returnObject(ftpClient);
}
}
}
```
阅读全文
相关推荐
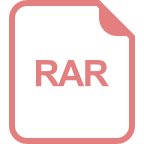


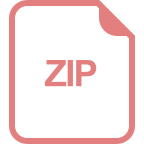
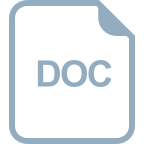



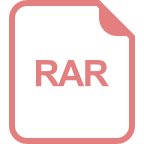
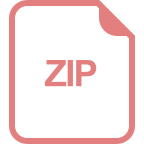
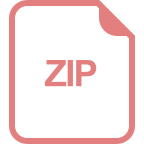
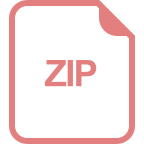
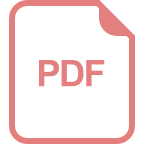
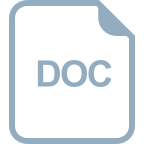
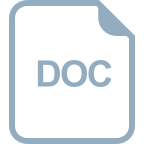
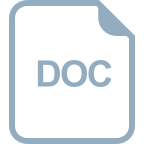
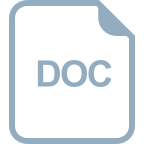