从一个数组中找出三个数,其和为180的所有可能,c++程序该怎么做
时间: 2024-10-16 18:07:40 浏览: 5
要在 C++ 中编写一个程序,用于找到给定数组中所有和为180的三个数,可以采用哈希集合(unordered_set)来存储已经遍历过的元素,避免重复。下面是一个简单的算法示例:
```cpp
#include <iostream>
#include <unordered_set>
#include <vector>
using namespace std;
void findThreeNumbers(vector<int>& nums, int target) {
unordered_set<int> hashSet;
for (int i = 0; i < nums.size(); i++) {
int complement = target - nums[i];
if (hashSet.find(complement) != hashSet.end()) {
cout << "Found a combination: (" << nums[i] << ", " << complement << ", " << target - nums[i] << ")" << endl;
}
hashSet.insert(nums[i]);
}
}
int main() {
vector<int> nums = {2, 7, 11, 15, 9};
int target = 180;
findThreeNumbers(nums, target);
return 0;
}
```
在这个程序中:
- `findThreeNumbers` 函数接受一个整数向量 `nums` 和目标和 `target`。
- 遍历数组,对于每个元素 `nums[i]`,计算其补数(即 `target - nums[i]`),检查这个补数是否已经在哈希集中,如果存在,则找到了一组和为180的三个数。
- 使用 `hashSet.insert(nums[i])` 将当前元素添加到哈希集合中,以便后续比较。
运行此程序会输出所有满足条件的三元组组合。例如,对于输入 `[2, 7, 11, 15, 9]`,结果可能是 `(9, 7, 10)` 或 `(2, 9, 18)` 等。
阅读全文
相关推荐
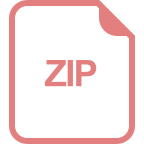
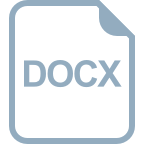
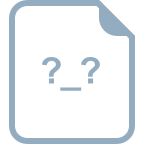















