echo -e "AT+QGPSCFG="outport","usbnmea"\n\r" > /dev/ttyUSB1
时间: 2024-06-05 15:05:43 浏览: 146
这是一条 AT 命令,用于配置 SIM7600 模块的 GPS 输出口为 USB NMEA 数据格式。具体解释如下:
- "AT+QGPSCFG=":表示发送 GPS 配置命令。
- "outport":表示 GPS 输出口类型,可以是 outport 或者 inport。outport 表示将 GPS 数据输出到一个外部设备,而 inport 则表示将外部设备的 GPS 数据输入到模块中。
- "usbnmea":表示 GPS 数据输出格式,此处为 USB NMEA 数据格式,也可以选择其他格式。
- "\n\r":表示换行回车符,表示一条 AT 命令的结束。
如果您需要使用此命令,请先确认您连接的设备是正确的,并且您已经获取了相应的 AT 命令权限。
相关问题
请帮我把我的代码进一步模块化:module sap1(outport,rst,cp); output [7:0]outport; input rst; input cp; parameter s0=3'b000, s1=3'b001, s2=3'b010, s3=3'b011, s4=3'b100, s5=3'b101; reg[2:0]pstate=3'b000; reg[2:0]nstate; reg[3:0]pc; reg[3:0]mar; reg[7:0]acc; reg[7:0]ir; reg[3:0]tmp; reg[7:0]breg; reg[7:0]outreg; reg run; wire cs; wire[7:0]romdata; wire[3:0]addrbus; wire[7:0]databus; reg flag,f1; reg[7:0]num; always@(negedge cp or posedge rst) begin if(rst) begin pc<=4'b0000; acc<=8'b0000_0000; run<=1'b1; pstate<=s0; nstate<=s0; flag=1; end else begin if(run) begin case(pstate) s0:begin nstate<=s1; f1=1; mar<=pc; end s1:begin nstate<=s2; if(flag) begin pc<=pc+1'b1; flag=1'b0; end end s2:begin nstate<=s3; flag=1; ir<=databus; end s3:begin nstate<=s4; tmp<=ir[7:4]; end s4:nstate<=s5; s5:nstate<=s0; endcase end if(pstate==s3) begin if((tmp==4'b0000)||(tmp==4'b0001)||(tmp==4'b0010)) mar<=ir[3:0]; else if(tmp==4'b1110) outreg<=acc; else if(tmp==4'b1111) run<=1'b0; end else if(pstate==s4) begin if(tmp==4'b0000) acc<=databus; else if(tmp==4'b0001) breg<=databus; else if(tmp==4'b0010) breg<=databus; end else if(pstate==s5) begin if((tmp==4'b0001)&&(f1==1'b1)) begin num=acc+breg; acc<=num; f1=1'b0; end else if((tmp==4'b0010)&&(f1==1'b1)) begin num=acc-breg; acc<=num; f1=1'b0; end end end end rom1 u1(romdata,addrbus,cs); assign addrbus=((pstate==s2)||(pstate==s4))?mar:4'b0000; assign databus=(cs==1'b0)?romdata:8'bxxxx_xxxx; assign cs=((pstate==s2)||(pstate==s4))?1'b0:1'b1; assign outport=outreg; endmodule
Sure! Here's a possible modularization of your code:
1. Control unit module:
```
module control_unit(input clk, input rst, output reg[2:0] pstate);
// Define states as parameters
parameter s0=3'b000, s1=3'b001, s2=3'b010, s3=3'b011, s4=3'b100, s5=3'b101;
// Internal signals
reg[2:0] nstate;
reg flag, f1;
reg[3:0] pc;
reg[3:0] mar;
reg[7:0] ir;
reg[3:0] tmp;
reg run;
// Outputs
output reg cs;
output reg[3:0] addrbus;
// Initialize state and flag
always @(posedge clk, posedge rst) begin
if (rst) begin
pstate <= s0;
flag <= 1'b1;
end else begin
pstate <= nstate;
end
end
// State machine
always @(*) begin
case (pstate)
s0: begin
nstate = s1;
f1 = 1;
mar = pc;
end
s1: begin
nstate = s2;
if (flag) begin
pc = pc + 1'b1;
flag = 1'b0;
end
end
s2: begin
nstate = s3;
flag = 1;
end
s3: begin
nstate = s4;
tmp = ir[7:4];
end
s4: begin
nstate = s5;
end
s5: begin
nstate = s0;
end
endcase
end
// Output logic
always @(*) begin
case (pstate)
s2, s4: begin
addrbus = mar;
cs = 1'b0;
end
default: begin
addrbus = 4'b0000;
cs = 1'b1;
end
endcase
end
endmodule
```
2. ALU module:
```
module alu(input clk, input rst, input [7:0] a, input [7:0] b, input [3:0] op, output reg[7:0] outreg);
// Internal signals
reg[7:0] num;
reg f1;
// Initialize flag
always @(posedge clk, posedge rst) begin
if (rst) begin
f1 <= 1'b1;
end else begin
f1 <= 1'b0;
end
end
// ALU operations
always @(*) begin
case (op)
4'b0000: begin // load a
outreg = a;
end
4'b0001: begin // load b
outreg = b;
end
4'b0010: begin // store b
outreg = b;
end
4'b0001, 4'b0010: begin // add/subtract with carry
if (f1) begin
num = a + b;
outreg = num;
f1 = 1'b0;
end
end
endcase
end
endmodule
```
3. Memory module:
```
module memory(input clk, input rst, input [3:0] addr, input [7:0] din, output reg[7:0] dout);
// Memory array
reg[7:0] mem[16];
// Initialize memory
initial begin
mem[0] = 8'h00; // NOP
mem[1] = 8'h01; // LDA addr
mem[2] = 8'h02; // LDB addr
mem[3] = 8'h03; // STB addr
mem[4] = 8'h04; // ADD
mem[5] = 8'h05; // SUB
mem[14] = 8'hFE; // OUT
mem[15] = 8'hFF; // HLT
end
// Read/write operations
always @(posedge clk, posedge rst) begin
if (rst) begin
dout <= 8'b0000_0000;
end else begin
if (din != 8'bz) begin
mem[addr] <= din;
end
dout <= mem[addr];
end
end
endmodule
```
4. Top-level module:
```
module sap1(outport, rst, cp);
// Inputs
input rst;
input cp;
// Outputs
output [7:0] outport;
// Internal signals
wire cs;
wire[7:0] romdata;
wire[3:0] addrbus;
wire[7:0] databus;
// Instantiate modules
control_unit u1(cp, rst, pstate, nstate, flag, f1, pc, mar, ir, tmp, run, cs, addrbus);
memory u2(cp, rst, addrbus, databus, ir);
alu u3(cp, rst, acc, breg, tmp, outreg);
memory u4(cp, rst, addrbus, databus, outreg);
// Connect memory and databus
assign databus = (cs == 1'b0) ? romdata : 8'bzzzz_zzzz;
assign romdata = u2.dout;
// Connect top-level inputs/outputs
assign outport = u4.dout;
endmodule
```
Please note that this is just one possible way of modularizing your code. The actual design may depend on specific requirements and constraints.
MOV DX,OUTPORT1 OUT DX,AL
这段汇编代码的作用是将AL寄存器中的数据输出到OUTPORT1端口上。
首先,MOV指令将OUTPORT1的端口地址存储在DX寄存器中,准备进行输出操作。
然后,OUT指令将AL寄存器中的数据输出到DX所指向的端口上,即OUTPORT1。
因此,这段汇编代码的功能是将AL寄存器中的数据输出到OUTPORT1端口上,实现了CPU与外部设备之间的数据交互。
阅读全文
相关推荐
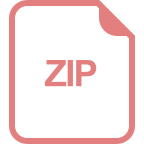
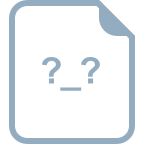
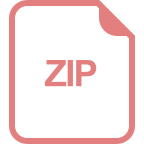
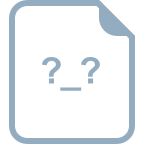
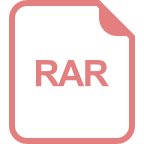
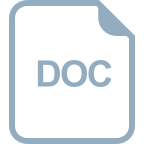
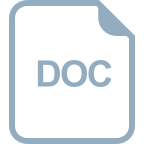
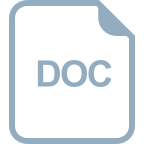
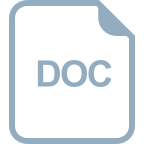



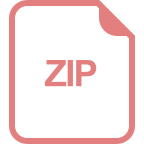
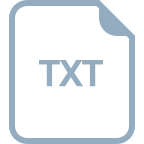