如何用C语言编程来实现牛顿迭代法求解函数零点?
时间: 2024-10-21 18:08:10 浏览: 40
牛顿迭代法是一种数值分析技术,用于找到函数的根(即零点)。在C语言中,你可以按照以下步骤实现:
1. **函数定义**:首先,你需要编写一个函数来表示你要找零点的那个函数f(x)及其导数f'(x)。
```c
double f(double x);
double df(double x);
```
2. **初始猜测值**:选择一个初始近似值x0作为函数零点的估计值。
3. **迭代循环**:在一个while循环中,计算下一个近似值x_new,公式是:\( x_{new} = x_{old} - \frac{f(x_{old})}{df(x_{old})} \)。
```c
double x_old = ...; // 初始值
double x_new;
do {
x_new = x_old - f(x_old) / df(x_old);
// 如果收敛条件满足(例如 |x_new - x_old| < ε 或者迭代次数达到最大限制),跳出循环
} while (!converged || ++iteration_count > max_iterations);
```
4. **结果检查**:当迭代收敛或达到最大迭代次数时,返回x_new作为近似的零点。
5. **错误处理**:考虑到实际计算可能存在浮点精度误差,你可以添加一些错误检查和处理机制。
注意:这里的`converged`、`ε`、`max_iterations`和`iteration_count`需要根据实际应用自行设定。
相关问题
C语言牛顿法求非线性方程的根,使用牛顿迭代法求解非线性方程的根
好的,我可以为您讲解一下C语言中使用牛顿迭代法求解非线性方程的根。
牛顿迭代法是一种通过不断逼近函数零点的方法,来求解非线性方程的根的方法。其基本思想是:在当前点处,用一条切线代替曲线,然后求解切线和x轴的交点,将此交点作为新的迭代点,如此往复,直到满足一定的精度要求为止。
下面是C语言中使用牛顿迭代法求解非线性方程的根的示例代码:
```c
#include <stdio.h>
#include <math.h>
#define EPS 1e-6
double f(double x) {
return x * x - 2; // 需要求解的非线性方程
}
double df(double x) {
return 2 * x; // 非线性方程的导函数
}
double newton(double x0) {
double x1 = x0;
while (fabs(f(x1)) > EPS) {
x1 = x0 - f(x0) / df(x0); // 牛顿迭代公式
x0 = x1;
}
return x1;
}
int main() {
double x0 = 2.0; // 初始值
double x = newton(x0);
printf("The root is: %lf\n", x);
return 0;
}
```
在上述代码中,`f`和`df`分别为需要求解的非线性方程和其导函数,`newton`函数则是使用牛顿迭代法求解非线性方程的根的函数。在`newton`函数中,我们设置了一个精度值`EPS`,当求解结果的绝对值小于该精度值时,就认为已经得到了一个较精确的解。
用C语言编写代码来实现“牛顿迭代法”求解方程 y = x 3 + e x y=x 3 +e x 的根
牛顿迭代法是一种数值优化算法,用于寻找函数零点。对于给定的一阶可导函数f(x),它通过不断逼近函数图像的切线与x轴的交点来逼近函数的根。以下是使用C语言实现牛顿迭代法的基本步骤:
```c
#include <stdio.h>
#include <math.h>
// 函数f(x) = x^3 + ex
double function(double x) {
return pow(x, 3) + exp(x);
}
// f'(x) 的导数
double derivative(double x) {
return 3 * pow(x, 2) + exp(x);
}
// 牛顿迭代法
double newton_raphson(double initial_guess, double tolerance, int max_iterations) {
double current_guess;
int iteration = 0;
do {
current_guess = initial_guess - function(initial_guess) / derivative(initial_guess);
if (fabs(function(current_guess)) < tolerance) { // 当函数值足够小满足条件停止迭代
break;
}
iteration++;
if (iteration == max_iterations) { // 达到最大迭代次数则返回最后猜测值
printf("达到最大迭代次数 (%d) ,无法找到更精确的解\n", max_iterations);
break;
}
} while (true);
return current_guess;
}
int main() {
double initial_guess = -2; // 初始猜测值
double tolerance = 1e-6; // 容许的误差范围
int max_iterations = 100; // 最大迭代次数
double root = newton_raphson(initial_guess, tolerance, max_iterations);
printf("方程的近似根为: %lf\n", root);
阅读全文
相关推荐
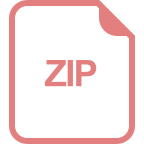
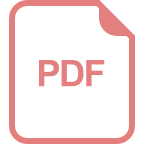
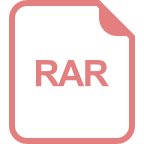













