用C语言编写代码来实现“牛顿迭代法”求解方程 y = x 3 + e x y=x 3 +e x 的根
时间: 2024-10-13 18:15:23 浏览: 78
牛顿迭代法是一种数值优化算法,用于寻找函数零点。对于给定的一阶可导函数f(x),它通过不断逼近函数图像的切线与x轴的交点来逼近函数的根。以下是使用C语言实现牛顿迭代法的基本步骤:
```c
#include <stdio.h>
#include <math.h>
// 函数f(x) = x^3 + ex
double function(double x) {
return pow(x, 3) + exp(x);
}
// f'(x) 的导数
double derivative(double x) {
return 3 * pow(x, 2) + exp(x);
}
// 牛顿迭代法
double newton_raphson(double initial_guess, double tolerance, int max_iterations) {
double current_guess;
int iteration = 0;
do {
current_guess = initial_guess - function(initial_guess) / derivative(initial_guess);
if (fabs(function(current_guess)) < tolerance) { // 当函数值足够小满足条件停止迭代
break;
}
iteration++;
if (iteration == max_iterations) { // 达到最大迭代次数则返回最后猜测值
printf("达到最大迭代次数 (%d) ,无法找到更精确的解\n", max_iterations);
break;
}
} while (true);
return current_guess;
}
int main() {
double initial_guess = -2; // 初始猜测值
double tolerance = 1e-6; // 容许的误差范围
int max_iterations = 100; // 最大迭代次数
double root = newton_raphson(initial_guess, tolerance, max_iterations);
printf("方程的近似根为: %lf\n", root);
阅读全文
相关推荐
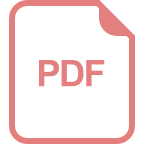
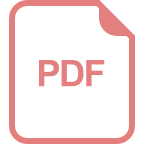
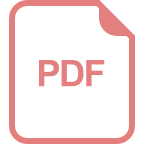















